How to Remove Specific Element from JSON Array in Javascript?
Nov 24, 2022 . Admin
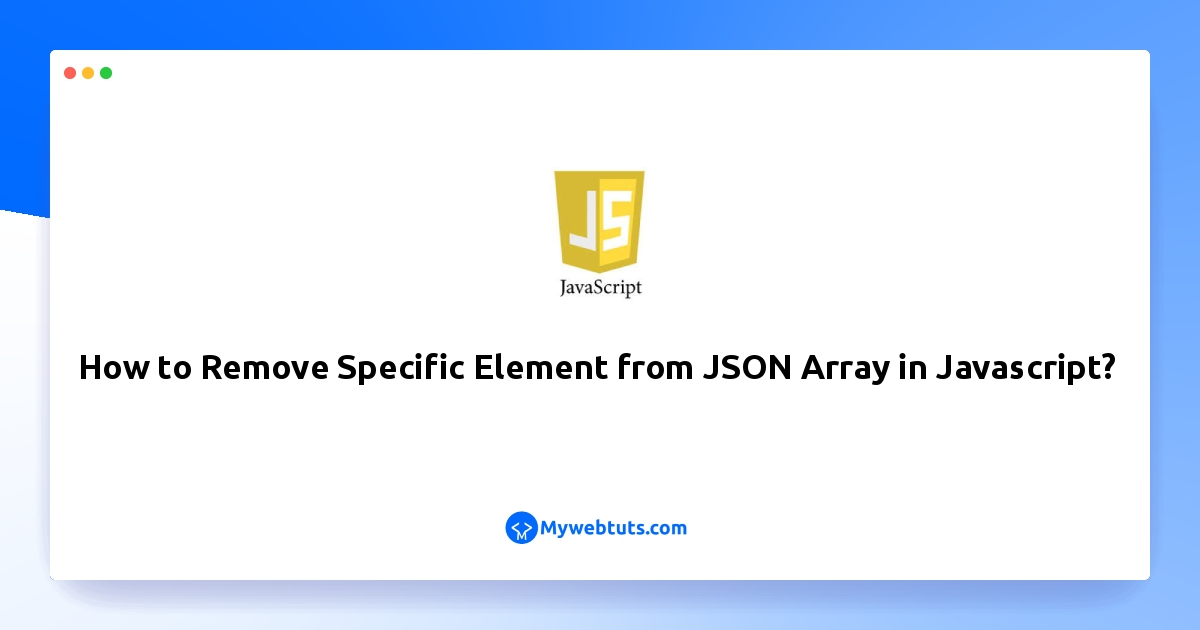
Hello friends,
This tutorial is focused on how to remove specific element from JSON in javascript. you can understand a concept of remove specific element from JSON array. I would like to show you how to remove a specific element from a JSON array with an example. you'll learn how to remove specific element from JSON array in javascript.
To remove the specific element from JSON array in javascript, we are required to write a JavaScript function that takes in one such array as the first argument and an id string as the second argument. Then our function should search for the object by that id, and if the array contains that object, we should remove it from the array.
So, let's see bellow solution:
Example<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>How to Remove Specific Element from JSON Array in Javascript?</title> </head> <body> <script type="text/javascript"> //create jsonArray const jsonArray = [ {id: "1", name: "Snatch", type: "crime"}, {id: "2", name: "Witches of Eastwick", type: "comedy"}, {id: "3", name: "X-Men", type: "action"}, {id: "4", name: "Ordinary People", type: "drama"}, {id: "5", name: "Billy Elliot", type: "drama"}, {id: "6", name: "Toy Story", type: "children"} ]; const removeById = (jsonArray, id) => { const requiredIndex = jsonArray.findIndex(el => { return el.id === String(id); }); if(requiredIndex === -1){ return false; }; return !!jsonArray.splice(requiredIndex, 1); }; //remove id:5 from jsonArray removeById(jsonArray, 5); //print jsonArray console.log(jsonArray); </script> </body> </html>Check The Console For Output:
0: {id: '1', name: 'Snatch', type: 'crime'} 1: {id: '2', name: 'Witches of Eastwick', type: 'comedy'} 2: {id: '3', name: 'X-Men', type: 'action'} 3: {id: '4', name: 'Ordinary People', type: 'drama'} 4: {id: '6', name: 'Toy Story', type: 'children'} length: 5
I hope it will help you...