How to Used String Helper Functions in Laravel 10
Nov 24, 2023 . Admin
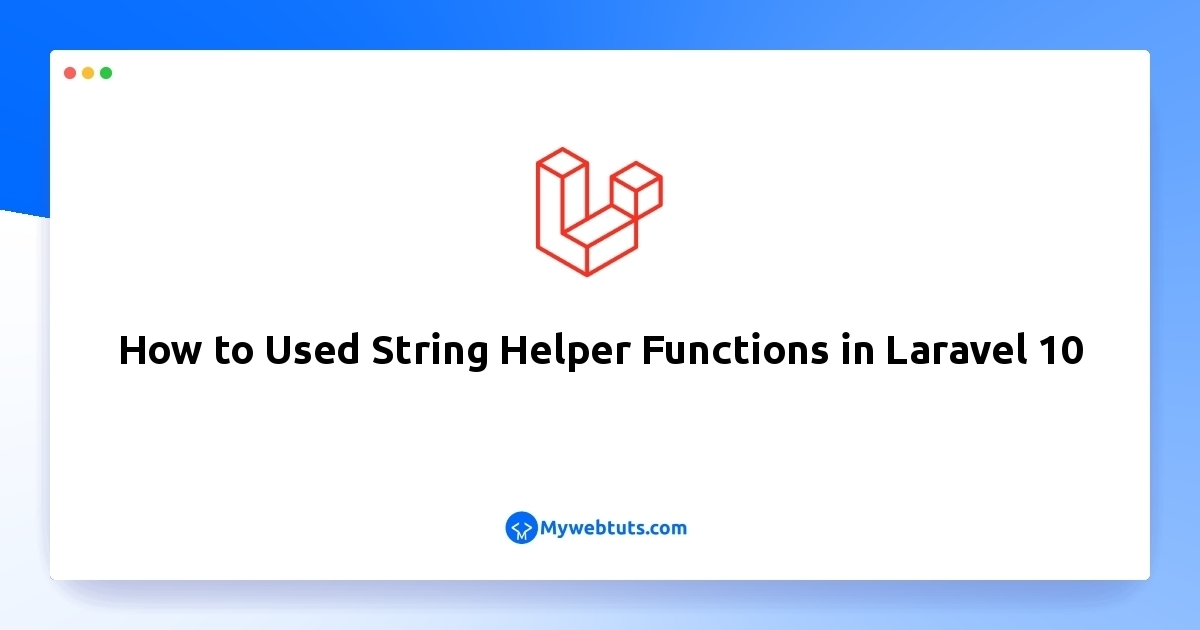
Hi dev,
Mastering string operations and manipulation is essential for any programmer, and Laravel provides a robust suite of helper functions to simplify these tasks. It's imperative for developers to stay abreast of the latest techniques and best practices to maximize the efficiency of these helper functions.
In this guide, we will delve into ten valuable tips for optimizing Laravel's string helper functions in the year 2023. Whether you're a novice or a seasoned Laravel developer, these tips will empower you to refine your string manipulation skills and optimize your code for efficiency.
Let's embark on a journey to unlock the full potential of Laravel's string helper functions.
Tip 1: Keep Laravel UpdatedEnsuring your Laravel framework is up-to-date brings various benefits, including improvements to string helper functions. New releases often introduce optimizations and enhancements that can significantly enhance your code's performance.
Tip 2: Harness the Str ClassThe Str class in Laravel offers a set of static methods for string manipulation. Leveraging this class helps organize your code and often leads to more optimized operations.
For instance:use Illuminate\Support\Str; $slug = Str::slug('Laravel String Helper Functions');Tip 3: Minimize Unnecessary Conversions
String and array conversions can impact performance. Whenever feasible, opt for helper functions that allow direct string operations to minimize unnecessary conversions.
For example:// Inefficient: Unnecessary conversion $string = "123"; $number = (int)$string; // Unnecessary conversion to integer // Efficient: Direct string operation $string = "123"; $number = $string; // Direct use without unnecessary conversionTip 4: Leverage Str::after and Str::before Functions
The Str::after and Str::before functions efficiently extract substrings based on specified delimiters.
$substring = Str::after('laravel.com', 'la'); // Returns 'ravel.com'Tip 5: Harness the Power of Regular Expressions
Regular expressions are potent tools for string manipulation. Laravel offers optimized functions that incorporate regular expressions for efficient operations.
use Illuminate\Support\Str; // Example 1: Extracting numbers from a string $string = "Laravel 10 is awesome! Version 9 was great too."; $numbers = preg_match_all('/\d+/', $string, $matches); // $matches will contain an array of matched numbers // ... (other examples omitted for brevity)Tip 6: Use Str::replaceArray Function
The Str::replaceArray function allows multiple replacements in a single call, providing performance benefits over using multiple str_replace calls.
use Illuminate\Support\Str; // Example: Replacing multiple placeholders in a string $template = "Hello, [name]! Today is [day]."; $replacements = ['John', 'Monday']; // Efficient: Using Str::replaceArray for multiple replacements $template = Str::replaceArray('[name]', $replacements, $template);Tip 7: Avoid Using explode and implode
Utilize Laravel's optimized functions for string splitting and joining instead of using explode and implode.
use Illuminate\Support\Str; // Example 1: Splitting a string into an array $string = "apple,orange,banana"; // Efficient: Using Str::of and explode $fruitsArray = Str::of($string)->explode(','); // Example 2: Joining elements of an array into a string $fruits = ['apple', 'orange', 'banana']; // Efficient: Using Str::of and implode $fruitString = Str::of($fruits)->implode(',');Tip 8: Embrace Str::random Function
The Str::random function generates random strings, useful for creating unique identifiers or passwords.
use Illuminate\Support\Str; // Example 1: Generating a random string with default length (16 characters) $randomString = Str::random(); // ... (other examples omitted for brevity)Tip 9: Utilize the Str::slug Function
The Str::slug function creates SEO-friendly URLs by converting spaces and special characters, offering a cleaner alternative to traditional string replacements.
use Illuminate\Support\Str; // Example 1: Creating a slug from a string $title = "Laravel 10 Release - What's New?"; $slug = Str::slug($title); // ... (other examples omitted for brevity)Tip 10: Avoid Using substr for Checking String Length
Instead of using substr for string length checks, employ the optimized Str::length function for efficient string length calculation.
use Illuminate\Support\Str; // Example 1: Checking string length with substr $text = "Hello, Laravel!"; // Efficient: Using Str::length to check string length $lengthWithStrLength = Str::length($text, 10); // ... (other examples omitted for brevity)Conclusion: Elevate Your Laravel Development
In conclusion, optimizing your Laravel 10 string helper functions is paramount for enhancing performance and code efficiency.
By adopting these ten tips, you'll streamline operations and ensure your application handles string manipulation with ease. Implement these practices to elevate your Laravel development experience in the year 2023.