Multiple Parameters Pass in Route Using Laravel Example
Jan 12, 2023 . Admin
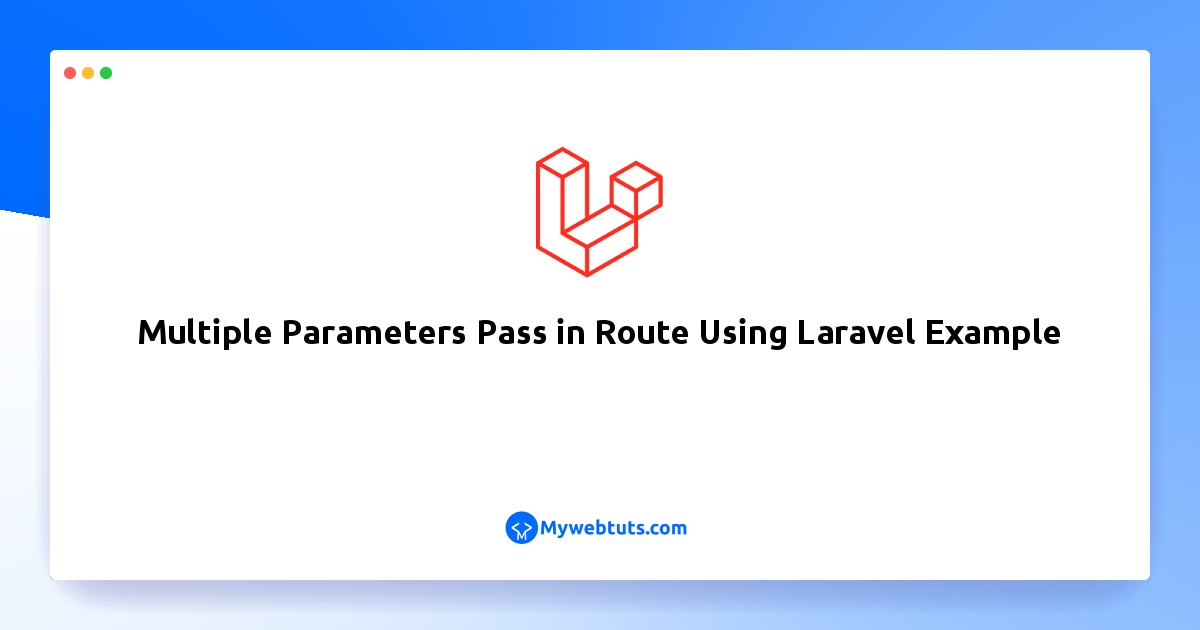
Hi Guys,
In this short tutorial, we'll show you how to utilise Laravel to provide various parameters to a route. In the Laravel tutorial, you'll learn how to use a variety of parameters. In this tutorial, we'll show you how to pass two arguments in a route in Laravel. In this post, you'll find a simple example of how to use several Laravel parameters in a route. Here, make a straightforward illustration of how to pass numerous arguments in a Laravel route.
Laravel Routes allows several arguments to be provided to the URL. The following two methods I'll share with you will inform the route to accept multiple parameters. Let's examine the following examples:
Example 1: Laravel Route Pass Multiple Parameters<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\UserController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('users/{user_id}/posts/{post_id}',[UserController::class, 'show'])->name("users.posts.show");App/Http/Controllers/UserController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class UserController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function show(Request $request, $user_id, $post_id) { dd($user_id, $post_id); } }Use It:
<a href="{{ route('users.posts.show', ['user_id' => 1, 'post_id' => 10]) }}">Show</a>Output:
1 10Example 2: Laravel Route Pass Multiple Parameters with Model Binding routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\UserController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('users/{user}/posts/{post}',[UserController::class, 'show'])->name("users.posts.show");App/Http/Controllers/UserController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use App\Models\Post; class UserController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function show(Request $request, User $user, Post $post) { dd($user->toArray(), $post->toArray()); return response()->json(['success'=>'User Updated Successfully!']); } }Use It:
<a href="{{ route('users.posts.show', ['user_id' => 1, 'post_id' => 10]) }}">Show</a>
.png)
I hope it can help you...