How To Check Two Numbers Are Approximately Equal In JavaScript?
Dec 06, 2021 . Admin
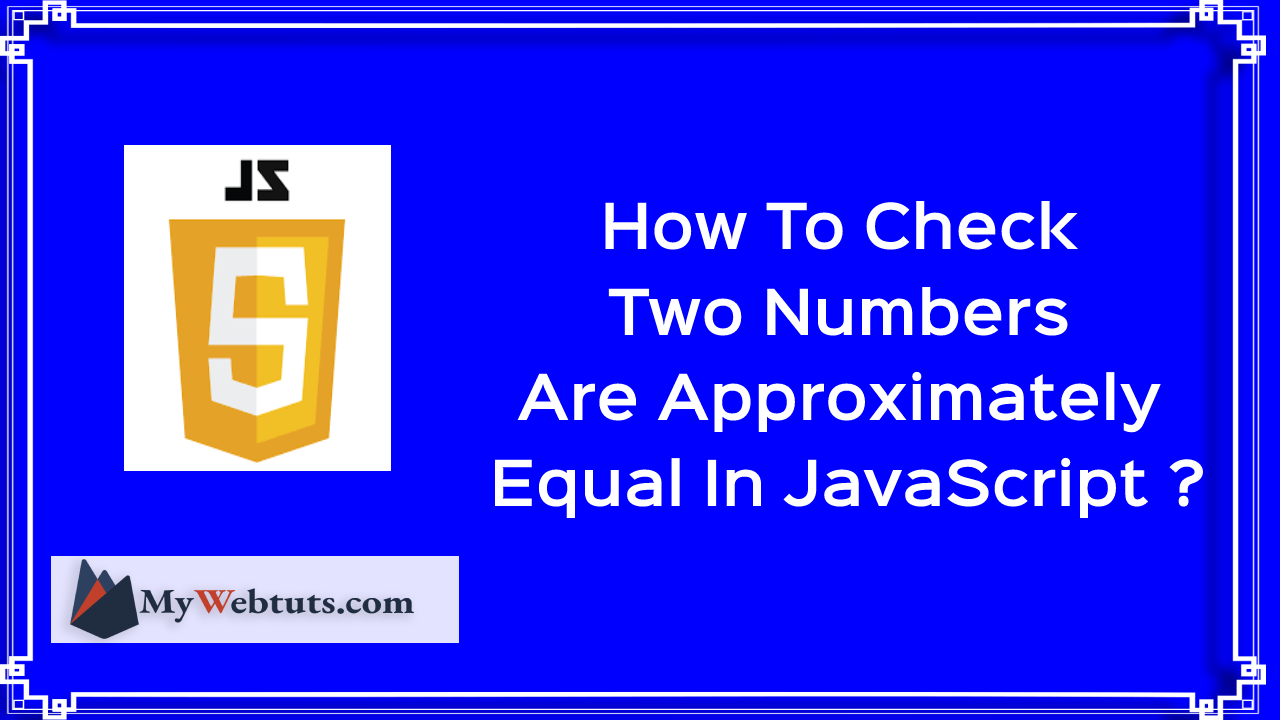
Hi Friend,
This is the example of check two numbers are approximately equal in JavaScript.
This is the short code and very easy example.
Given two numbers and the task is to check the given numbers are approximately equal to each other or not.
If both numbers are approximately the same then print true otherwise print false.
Example : 1<script> const checkApprox = (num1, num2, epsilon) => { // Calculating the absolute difference // and compare with epsilon return Math.abs(num1 - num2) < epsilon; } console.log(checkApprox(10.003, 10.001, 0.005)); </script>Output :
trueExample : 2
<script> const checkApprox = (num1, num2, epsilon = 0.004) => { return Math.abs(num1 - num2) < epsilon; } console.log(checkApprox(Math.PI / 2.0, 1.5708)); </script>Output :
trueExample : 3
<script> const checkApprox = (num1, num2, epsilon = 0.004) => { return Math.abs(num1 - num2) < epsilon; } console.log(checkApprox(0.003, 0.03)); </script>Output :
false