Laravel Registration Image Upload With an Example
Nov 30, 2022 . Admin
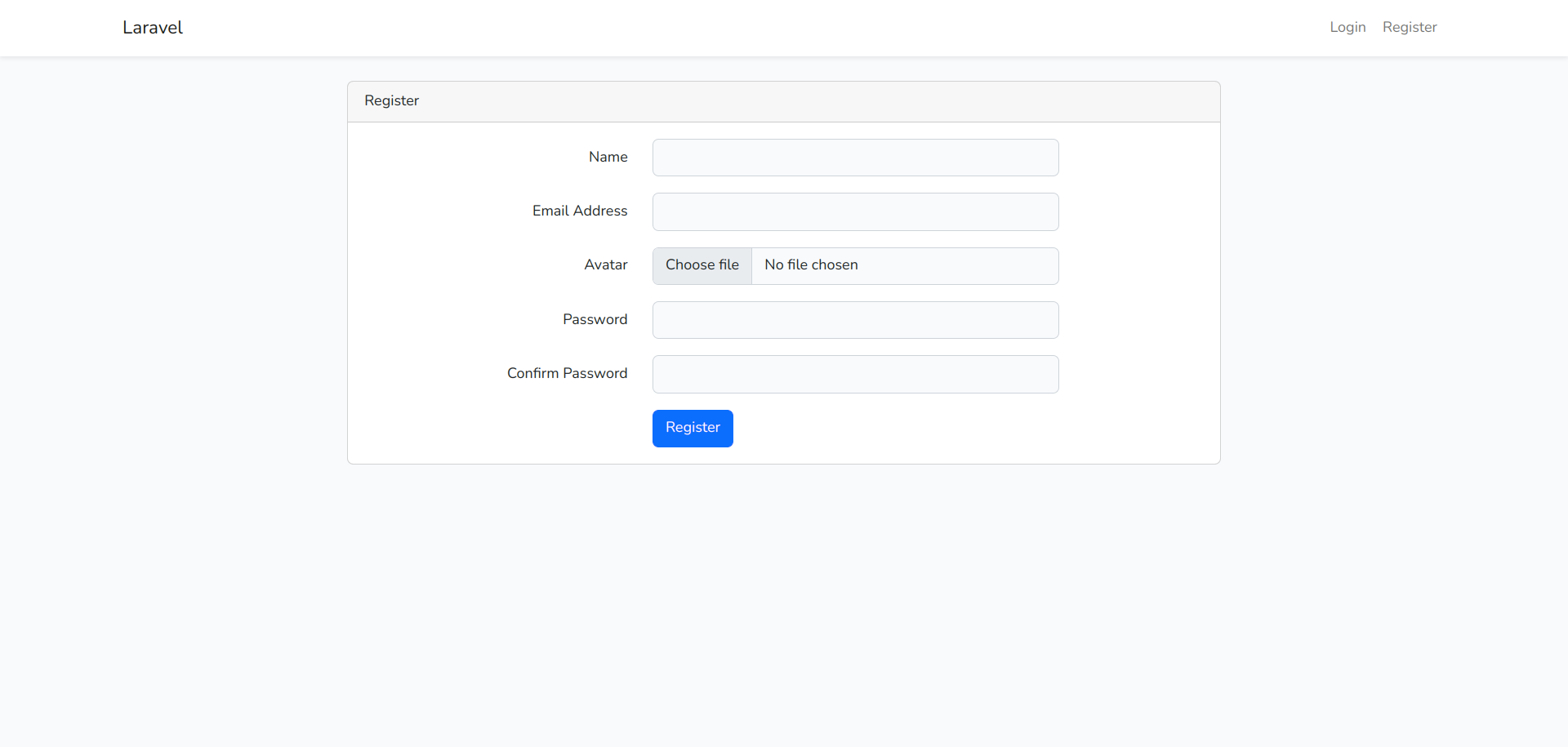
Hello Friends,
Here, I will show you the laravel registration image upload with an example. step by step explains laravel and how to upload profile images of users in laravel. if you want to see an example of uploading a profile picture in laravel registration then you are in the right place. I explained simply about the laravel avatar upload in the registration form. Here, Creating a basic example of how to upload images in the registration form in laravel.
If you want to upload pictures when the user registers in laravel. Then I will show you a step-by-step example of how to upload image in registration form in laravel. In this example, we will install laravel ui for user authentication. Then we will add a new column avatar to store the image path. So, let's see the below steps:
You can use this example with the versions of laravel 6, laravel 7, laravel 8, and laravel 9.
Step 1: Install LaravelThis is optional; however, if you have not created the laravel app, then you may go ahead and execute the below command:
composer create-project laravel/laravel example-appStep 2: Setup Database Configuration
After successfully installing the laravel app then after configuring the database setup. We will open the ".env" file and change the database name, username and password in the env file.
.envDB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=Enter_Your_Database_Name DB_USERNAME=Enter_Your_Database_Username DB_PASSWORD=Enter_Your_Database_PasswordStep 3: Install Auth Scaffold
Laravel's laravel/ui package provides a quick way to scaffold all of the routes and views you need for authentication using a few simple commands:
composer require laravel/ui
Next, we need to generate auth scaffold with bootstrap, so let's run the below command:
php artisan ui bootstrap --auth
Then, install npm packages using the below command:
npm install
At last, built bootstrap CSS using the below command:
npm run buildStep 4: Create Migration
In this step, we need to create new migration to add avatar field to users table. so let's run the below code and run migration.
php artisan make:migration add_new_fields_usersdatabase/migrations/2022_11_24_110854_add_new_fields_users.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::table('users', function (Blueprint $table) { $table->string('avatar')->nullable(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::table('users', function (Blueprint $table) { $table->dropColumn('avatar'); }); } };
Now, run migration with following command:
php artisan migrate
Next, update User.php model file.
app/Models/User.php<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Laravel\Sanctum\HasApiTokens; class User extends Authenticatable { use HasApiTokens, HasFactory, Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', 'avatar', ]; /** * The attributes that should be hidden for serialization. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; }Step 5: Update Blade File
In this step, we will update register blade file. in this file we will add file input for profile picture upload. so let's update following file:
resources/views/auth/register.blade.php@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">{{ __('Register') }}</div> <div class="card-body"> <form method="POST" action="{{ route('register') }}" enctype="multipart/form-data"> @csrf <div class="row mb-3"> <label for="name" class="col-md-4 col-form-label text-md-end">{{ __('Name') }}</label> <div class="col-md-6"> <input id="name" type="text" class="form-control @error('name') is-invalid @enderror" name="name" value="{{ old('name') }}" required autocomplete="name" autofocus> @error('name') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="email" class="col-md-4 col-form-label text-md-end">{{ __('Email Address') }}</label> <div class="col-md-6"> <input id="email" type="email" class="form-control @error('email') is-invalid @enderror" name="email" value="{{ old('email') }}" required autocomplete="email"> @error('email') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="avatar" class="col-md-4 col-form-label text-md-end">{{ __('Avatar') }}</label> <div class="col-md-6"> <input id="avatar" type="file" class="form-control @error('avatar') is-invalid @enderror" name="avatar" value="{{ old('avatar') }}" required autocomplete="avatar"> @error('avatar') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="password" class="col-md-4 col-form-label text-md-end">{{ __('Password') }}</label> <div class="col-md-6"> <input id="password" type="password" class="form-control @error('password') is-invalid @enderror" name="password" required autocomplete="new-password"> @error('password') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="password-confirm" class="col-md-4 col-form-label text-md-end">{{ __('Confirm Password') }}</label> <div class="col-md-6"> <input id="password-confirm" type="password" class="form-control" name="password_confirmation" required autocomplete="new-password"> </div> </div> <div class="row mb-0"> <div class="col-md-6 offset-md-4"> <button type="submit" class="btn btn-primary"> {{ __('Register') }} </button> </div> </div> </form> </div> </div> </div> </div> </div> @endsectionStep 6: Update RegisterController Controller
Here, we will update RegisterController with validator() and create() method. image will store in avatars folder in public. so let's copy the below code and add to controller file:
app/Http/Controllers/Auth/RegisterController.php<?php namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use App\Providers\RouteServiceProvider; use App\Models\User; use Illuminate\Foundation\Auth\RegistersUsers; use Illuminate\Support\Facades\Hash; use Illuminate\Support\Facades\Validator; class RegisterController extends Controller { /* |-------------------------------------------------------------------------- | Register Controller |-------------------------------------------------------------------------- | | This controller handles the registration of new users as well as their | validation and creation. By default this controller uses a trait to | provide this functionality without requiring any additional code. | */ use RegistersUsers; /** * Where to redirect users after registration. * * @var string */ protected $redirectTo = RouteServiceProvider::HOME; /** * Create a new controller instance. * * @return void */ public function __construct() { $this->middleware('guest'); } /** * Get a validator for an incoming registration request. * * @param array $data * @return \Illuminate\Contracts\Validation\Validator */ protected function validator(array $data) { return Validator::make($data, [ 'name' => ['required', 'string', 'max:255'], 'avatar' => ['image'], 'email' => ['required', 'string', 'email', 'max:255', 'unique:users'], 'password' => ['required', 'string', 'min:8', 'confirmed'], ]); } /** * Create a new user instance after a valid registration. * * @param array $data * @return \App\Models\User */ protected function create(array $data) { if(request()->hasfile('avatar')){ $avatarName = time().'.'.request()->avatar->getClientOriginalExtension(); request()->avatar->move(public_path('avatars'), $avatarName); } return User::create([ 'name' => $data['name'], 'email' => $data['email'], 'avatar' => $avatarName ?? NULL, 'password' => Hash::make($data['password']), ]); } }Run Laravel App:
All the required steps have been done, now you have to type the given below command and hit enter to run the Laravel app:
php artisan serve
Now, Go to your web browser, type the given URL and view the app output:
http://localhost:8000/Output:
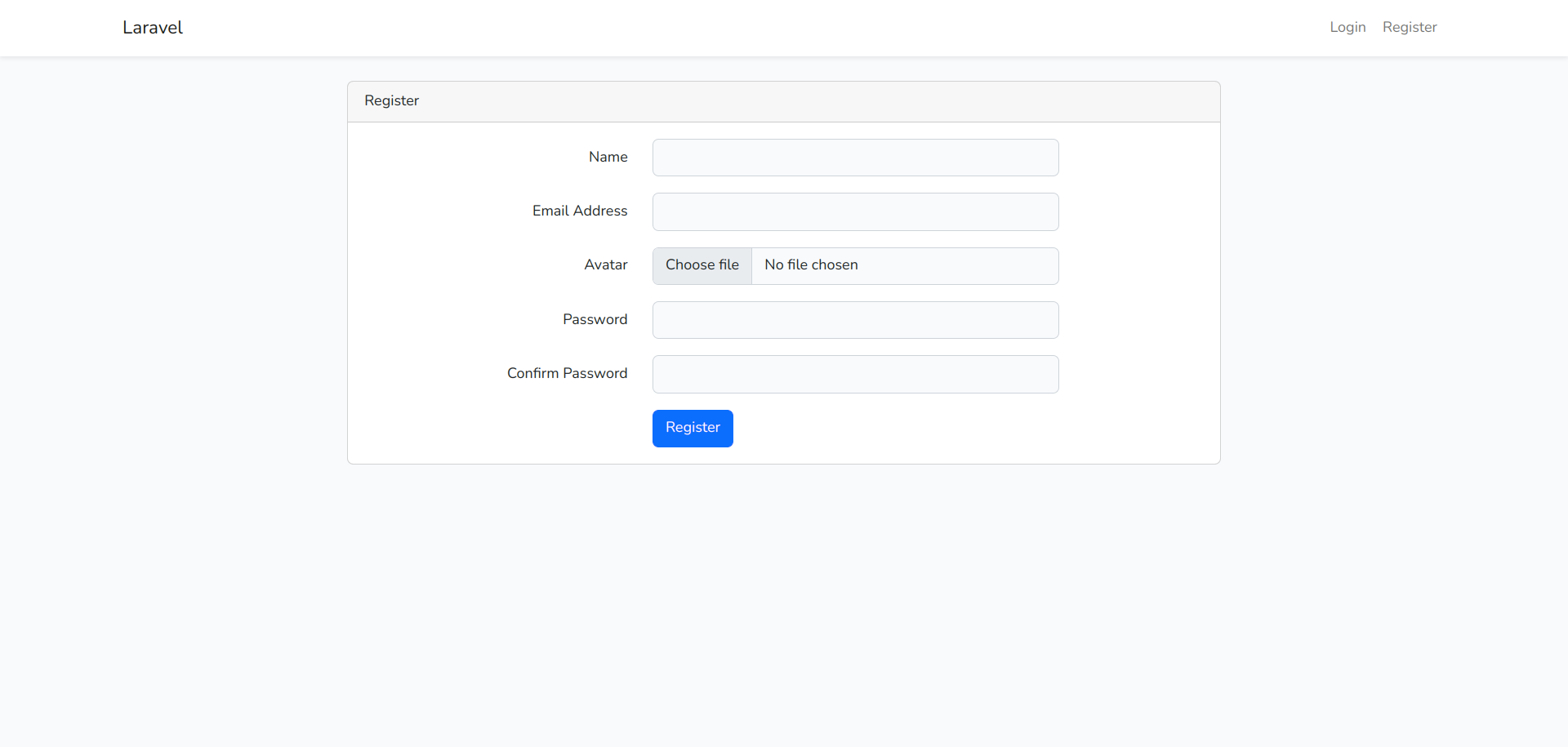
I hope it can help you...