PHP Ajax Image Upload with Preview Example
Mar 12, 2022 . Admin
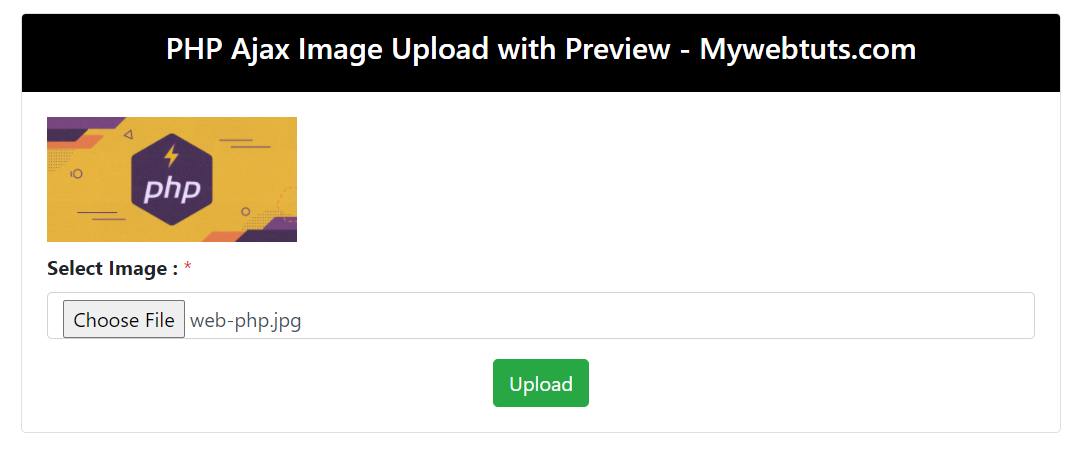
Hello Friends,
I am going to explain you PHP Ajax image upload with preview example. You will learn how to image upload and preview in PHP with Ajax. In side this article we will see how to Ajax image upload using PHP, jQuery with image Preview.
This article will give you simple example of jQuery ajax image upload in PHP with preview. We will use get simple preview an image before uploading using PHP with ajax.
You can use from how to PHP with Ajax code for image upload and display. I will give you simple how to image preview and upload using PHP with Ajax.
So, let's see bellow solution:
index.php<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>PHP MySQL AJAX Image Upload - Mywebtuts.com</title> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/css/bootstrap.min.css" integrity="sha384-zCbKRCUGaJDkqS1kPbPd7TveP5iyJE0EjAuZQTgFLD2ylzuqKfdKlfG/eSrtxUkn" crossorigin="anonymous"> </head> <body> <div class="container mt-5"> <div class="row"> <div class="col-md-12"> <div class="card w-75 m-auto"> <div class="card-header text-center text-white" style="background: black;"> <h4>PHP Ajax Image Upload with Preview - Mywebtuts.com</h4> </div> <div class="card-body"> <form action="upload.php" id="form" method="post" enctype="multipart/form-data"> <img id="preview-image" width="200px" class="mb-2"> <div class="form-group"> <label for="myImage"><strong>Select Image : </strong><span class="text-danger">*</span></label> <input type="file" id="myImage" class="form-control"> <p id="errorMs" class="text-danger"></p> </div> <div class="d-flex justify-content-center"> <input type="submit" class="btn btn-success" id="submit" value="Upload"> </div> </form> </div> </div> </div> </div> </div> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script> $(document).ready(function(){ $('#myImage').change(function(){ let reader = new FileReader(); reader.onload = (e) => { $('#preview-image').attr('src', e.target.result); $("#errorMs").hide(); } reader.readAsDataURL(this.files[0]); }); $("#submit").click(function(e){ e.preventDefault(); let form_data = new FormData(); let img = $("#myImage")[0].files; if(img.length > 0){ form_data.append('my_image', img[0]); $.ajax({ url: 'upload.php', type: 'post', data: form_data, contentType: false, processData: false, success: function(res){ const data = JSON.parse(res); if (data.error != 1) { $('.form-group').prepend('<p class="alert alert-success">Upload Successfully.</p>') }else { $("#errorMs").text(data.em); } $('#preview-image').fadeOut(800); } }); }else { $("#errorMs").text("Please select an image."); } }); }); </script> </body> </html>upload.php
<?php if (isset($_FILES['my_image'])) { $img_name = $_FILES['my_image']['name']; $img_size = $_FILES['my_image']['size']; $tmp_name = $_FILES['my_image']['tmp_name']; $error = $_FILES['my_image']['error']; if ($error === 0) { if ($img_size > 1000000) { $em = "Sorry, your file is too large."; $error = array('error' => 1, 'em'=> $em); echo json_encode($error); exit(); }else { $img_ex = pathinfo($img_name, PATHINFO_EXTENSION); $img_ex_lc = strtolower($img_ex); $allowed_exs = array("jpg", "jpeg", "png"); if (in_array($img_ex_lc, $allowed_exs)) { $new_img_name = uniqid("IMG-", true).'.'.$img_ex_lc; $img_upload_path = "uploads/".$new_img_name; move_uploaded_file($tmp_name, $img_upload_path); $res = array('error' => 0, 'src'=> $new_img_name); echo json_encode($res); exit(); }else { $em = "You can't upload files of this type"; $error = array('error' => 1, 'em'=> $em); echo json_encode($error); exit(); } } }else { $em = "unknown error occurred!"; $error = array('error' => 1, 'em'=> $em); echo json_encode($error); exit(); } } ?>Output:
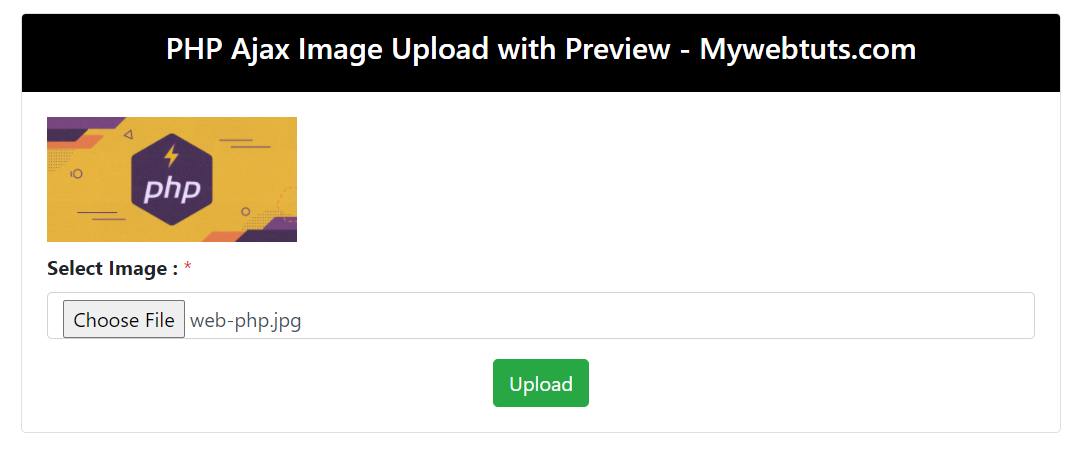
It will help you...