React JS Axios Post Request Example
Aug 29, 2022 . Admin
Hello Dev,
This tutorial shows you react js axios post request example. you can understand a concept of react js axios post example. This article will give you a simple example of react.js axios post example. if you want to see an example of react axios post json example then you are in the right place. So, let us dive into the details.
In this example, i will show you how to make Axios Post Request in React JS App. we will install axios using npm command. Then we will use "jsonplaceholder" demo api for post request. we will create simple form with name input and add submit button to call post request.
So, let's see follow the below step to create simple example with axios post request.
Step 1: Create React JS AppIn this step, open your terminal and execute the following command on your terminal to create a new react app:
npx create-react-app react-axios-exampleStep 2: Install Axios npm Package
In this step, we need to install axios npm package to use their functions. let's run below command:
npm install axiosStep 3: Install Bootstrap
In this step, we will install bootstrap npm package to use design css. let's run below command:
npm install bootstrap --saveStep 4: Create User.js Component
Here, we will create User.js component and make form with input text. Then call axios post request by clicking on add button. so let's update code on following file.
src/User.jsimport React from 'react'; import axios from 'axios'; class User extends React.Component { state = { name: '', } handleChange = event => { this.setState({ name: event.target.value }); } handleSubmit = event => { event.preventDefault(); const user = { name: this.state.name }; axios.post(`https://jsonplaceholder.typicode.com/users`, { user }) .then(res => { console.log(res); console.log(res.data); }) } render() { return ( <div> <form onSubmit={this.handleSubmit}> <label> Name: <input type="text" name="name" onChange={this.handleChange} /> </label> <button type="submit" class="btn btn-success">Add</button> </form> </div> ) } } export default User;Step 5: Update App.js File
Here, we will update our App.js file code. we will import User component and import bootstrap for design. so let's update code on App.js file.
src/App.jsimport './App.css'; import '../node_modules/bootstrap/dist/css/bootstrap.min.css'; import User from './User'; function App() { return ( <div class="container"> <h2>React JS Axios Post Request Example - MyWebTuts.com</h2> <User /> </div> ); } export default App;Step 6: Run React JS App
In the last step run your project using bellow command.
npm startOutput
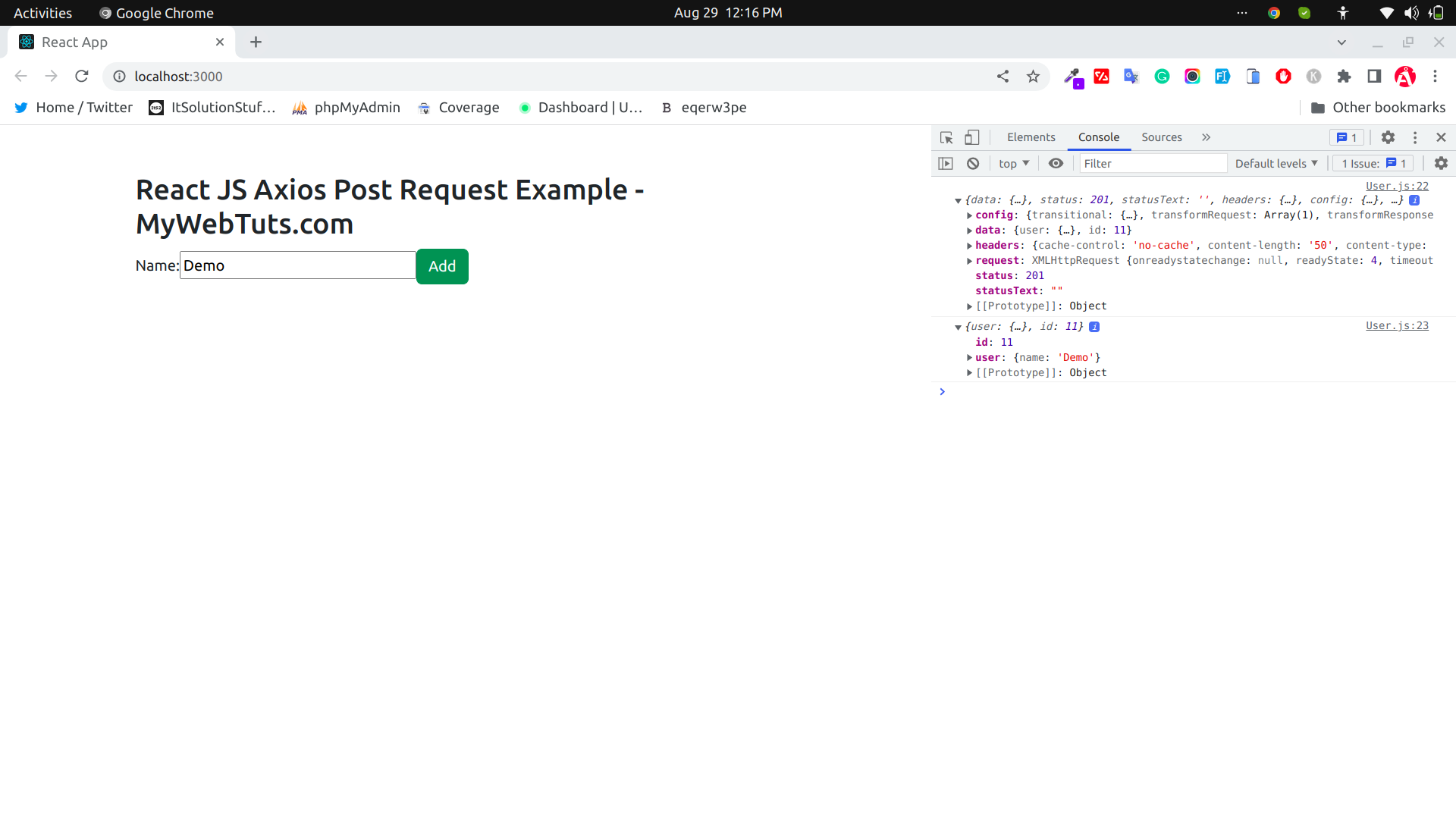
I hope it can help you...