Ajax Image Upload and Crop with Jquery and PHP Example
Mar 15, 2022 . Admin
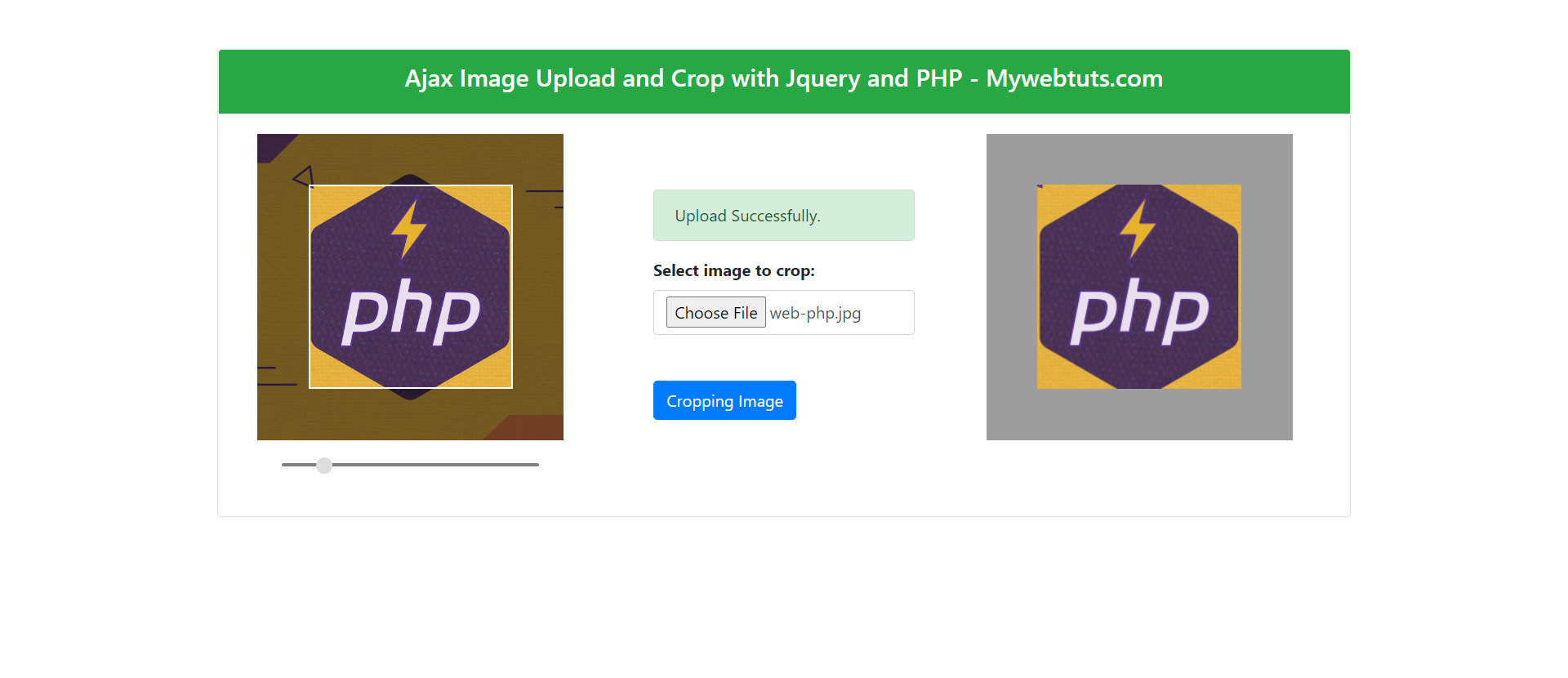
Hello dev,
I am going to explain you image crop and uploading using JQuery with PHP Ajax. You will learn how to upload and crop image using PHP and jQuery and Ajax. In side this article we will see how to Ajax image upload and crop with Jquery and PHP.
This article will give you simple example of how to crop image and upload PHP Ajax with Jquery. We will use get simple image upload and crop with Jquery Ajax and PHP.
I will give you simple Example how to image upload resize with Jquery Ajax and PHP so follow this and learn crop and save image using jquery Ajax and PHP.
So, let's see bellow solution:
index.php<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <title>Page Title</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.1.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/croppie/2.6.2/croppie.min.css"> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/croppie/2.6.2/croppie.js"></script> </head> <body> <div class="container mt-5"> <div class="card" style="max-height: 500px;"> <div class="card-header bg-success text-center text-white text-bold"> <h4>Ajax Image Upload and Crop with Jquery and PHP - Mywebtuts.com</h4> </div> <div class="card-body"> <div class="row"> <div class="col-md-4 text-center"> <div id="upload-demo"></div> </div> <div class="col-md-4" style="padding:5%;"> <div class="form-group"> <label><strong>Select image to crop:</strong></label> <input type="file" id="image" class="form-control" required> <span id="error" class="text-danger"></span><br> </div> <button class="btn btn-primary btn-upload-image" style="margin-top:2%">Cropping Image</button> </div> <div class="col-md-4"> <div id="preview-crop-image" style="background:#9d9d9d;width:300px;padding:50px 50px;height:300px;"></div> </div> </div> </div> </div> </div> <script> var resize = $('#upload-demo').croppie({ enableExif: true, enableOrientation: true, viewport: { // Default { width: 100, height: 100, type: 'square' } width: 200, height: 200, type: 'square' //square }, boundary: { width: 300, height: 300 } }); $("input").prop('required',true); $('#image').change(function () { var ext = this.value.match(/\.(.+)$/)[1]; switch (ext) { case 'jpg': case 'png': case 'jpeg': case 'pneg': $('#error').text(""); $('button').attr('disabled', false); break; default: $('#error').text("File must be of type jpg,png,pneg,jpeg."); $('button').attr('disabled', true); this.value = ''; } }); $('#image').on('change', function () { var reader = new FileReader(); reader.onload = function (e) { resize.croppie('bind',{ url: e.target.result }).then(function(){ console.log('jQuery bind complete'); }); } reader.readAsDataURL(this.files[0]); }); $('.btn-upload-image').on('click', function (ev) { resize.croppie('result', { type: 'canvas', size: 'viewport' }).then(function (img) { $.ajax({ url: "upload.php", type: "POST", data: {"image":img}, success: function (data) { html = '<img src="' + img + '" />'; $("#preview-crop-image").html(html); $('.form-group').prepend('<p class="alert alert-success">Upload Successfully.</p>') } }); }); }); </script> </body> </html>upload.php
<?php $image = $_POST['image']; list($type, $image) = explode(';',$image); list(, $image) = explode(',',$image); $image = base64_decode($image); $image_name = time().'.png'; file_put_contents('uploads/'.$image_name, $image); ?>Output:
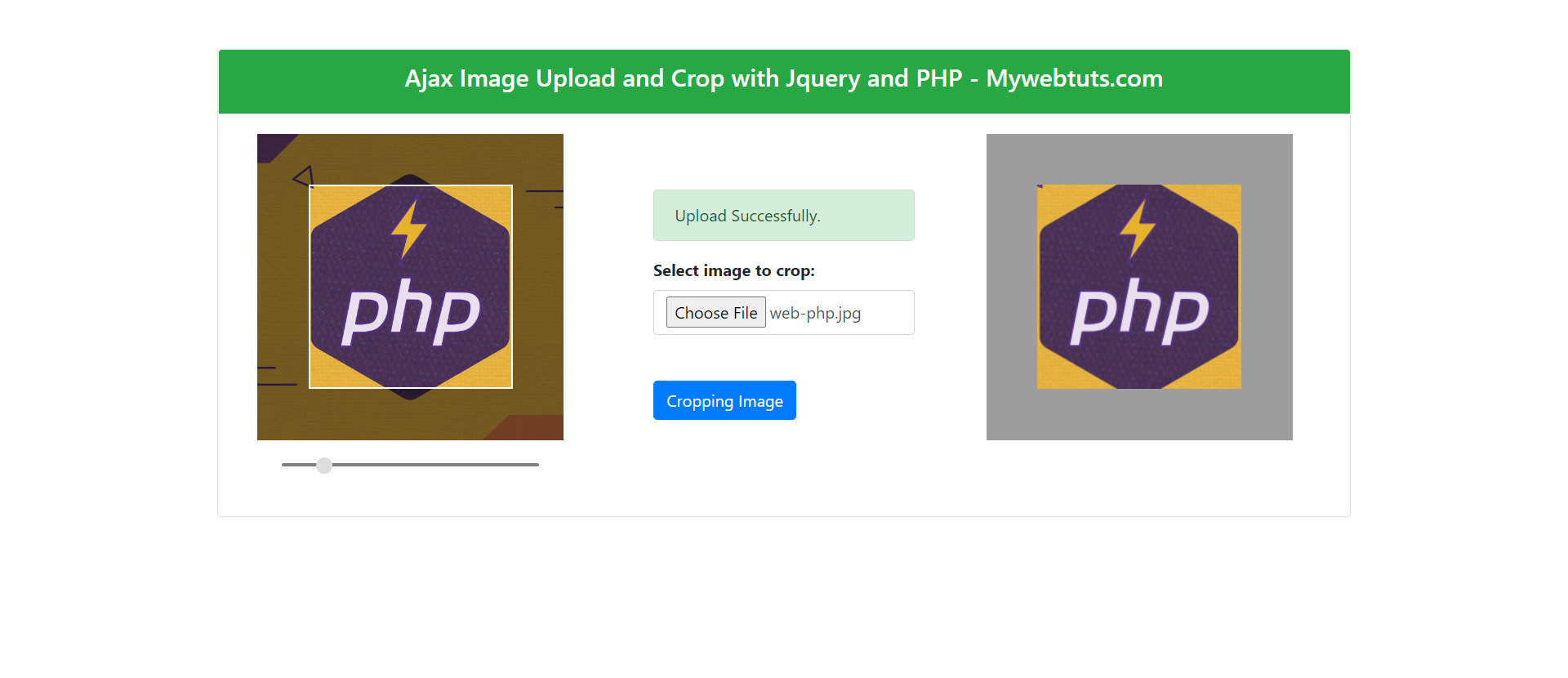
It will help you...