How to Delete Record using Ajax in PHP?
Mar 14, 2022 . Admin
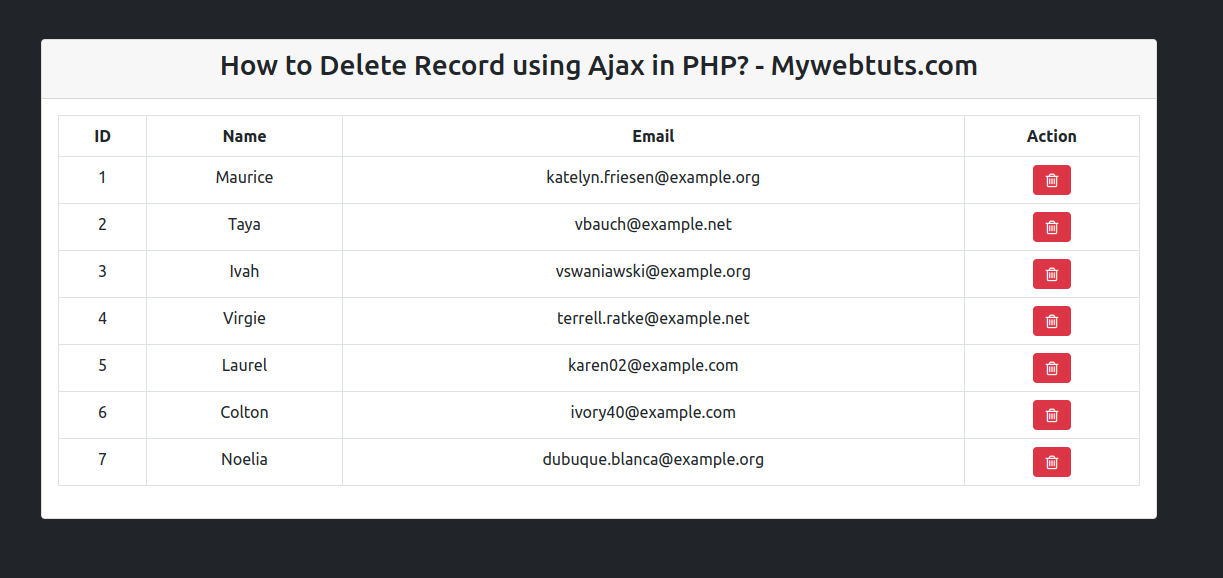
Hello dev,
I am going to explain you How to Delete Data From Database Using Ajax in PHP. You will learn how to delete row with ajax function and php - jquery. In side this article we will see how to Delete Record from MySQL Table with AJAX.
This article will give you simple example of Delete Data with jQuery in PHP & MySQL Using Ajax. We will use get simple Delete data using AJAX with PHP and MySQL.
I will give you simple Example how to Delete a table-row using JQuery Ajax without page reload.
So, let's see bellow solution:
config.php<?php $host = "localhost"; $user = "root"; $password = "root"; $dbname = "db_php"; $con = mysqli_connect($host, $user, $password,$dbname); if (!$con) { die("Connection failed: " . mysqli_connect_error()); } ?>index.php
<?php include "config.php"; ?> <!doctype html> <html> <head> <title>How to Delete Record using Ajax in PHP?</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css"> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" integrity="sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ==" crossorigin="anonymous" referrerpolicy="no-referrer"></script> </head> <body class="bg-dark"> <div class='container'> <div class="content mt-5"> <div class="card"> <div class="card-header"> <h3 class="text-center">How to Delete Record using Ajax in PHP? - Mywebtuts.com</h3> </div> <div class="card-body"> <table class="table text-center table-hover table-bordered"> <tr> <th>ID</th> <th>Name</th> <th>Email</th> <th>Action</th> </tr> <?php $query = "SELECT * FROM authors"; $result = mysqli_query($con,$query); $count = 1; while($row = mysqli_fetch_array($result) ){ $id = $row['id']; ?> <tr> <td align='center'><?= $count; ?></td> <td><?php echo $row['first_name'] ?></td> <td><?php echo $row['email'] ?></td> <td align='center'><span class='delete' data-id='<?= $id; ?>'><i class="fa fa-trash-o btn btn-danger" aria-hidden="true"></i></span></td> </tr> <?php $count++; } ?> </table> </div> </div> </div> </div> </body> <script type="text/javascript"> $(document).ready(function(){ $('.delete').click(function(){ var el = this; var deleteid = $(this).data('id'); var confirmalert = confirm("Are you sure?"); if (confirmalert == true) { $.ajax({ url: 'remove.php', type: 'POST', data: { id:deleteid }, success: function(response){ if(response == 1){ $(el).closest('tr').css('background','tomato'); $(el).closest('tr').fadeOut(800,function(){ $(this).remove(); }); }else{ alert('Invalid ID.'); } } }); } }); }); </script> </html>remove.php
<?php include "config.php"; $id = 0; if(isset($_POST['id'])){ $id = mysqli_real_escape_string($con,$_POST['id']); } if($id > 0){ // Check record exists $checkRecord = mysqli_query($con,"SELECT * FROM authors WHERE id=".$id); $totalrows = mysqli_num_rows($checkRecord); if($totalrows > 0){ // Delete record $query = "DELETE FROM authors WHERE id=".$id; mysqli_query($con,$query); echo 1; exit; }else{ echo 0; exit; } } echo 0; exit; ?>Output:
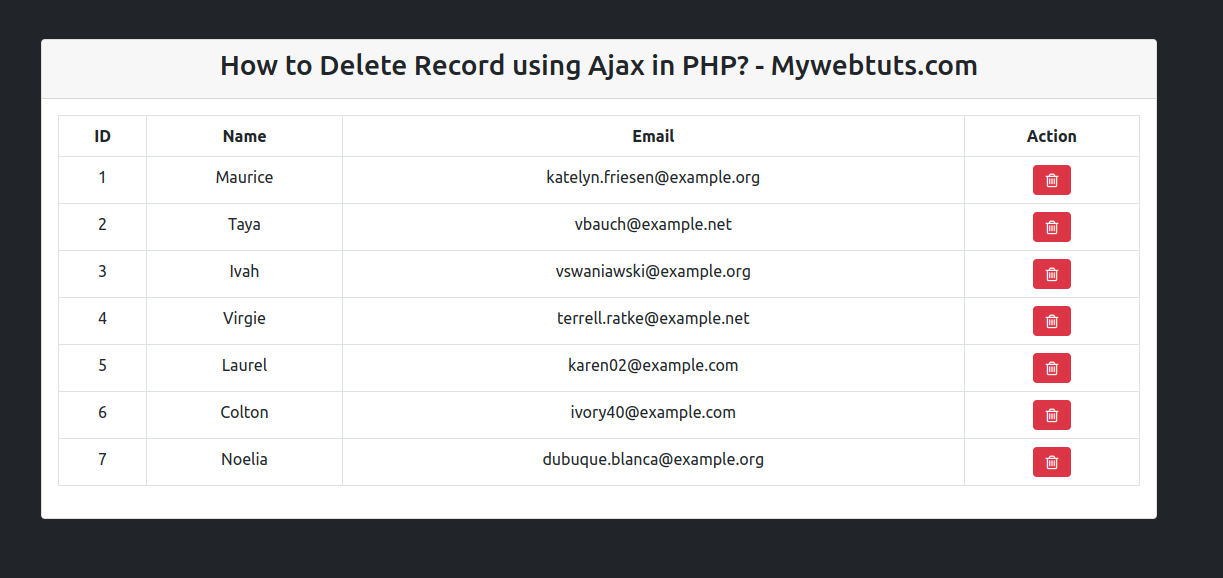
It will help you...