How to use Ajax Request in Laravel 9?
Feb 21, 2022 . Admin
Hi friends,
This article will provide some of the most important how to use ajax request in laravel 9?. This article will provide example of laravel 9 jquery ajax request example. you can see laravel 9 ajax request form submit example. In this article, we will implement a jquery ajax request laravel 9. this example will help you laravel 9 jquery ajax request example with store data in database.
Ajax request is a basic requirement of any php project, we are always looking for without page refresh data should store in database and it's possible only by jquery ajax request. same thing if you need to write ajax form submit in laravel 9 then i will help you how you can pass data with ajax request and get on controller.
Step 1: Download LaravelLet us begin the tutorial by installing a new laravel application. if you have already created the project, then skip following step.
composer create-project laravel/laravel example-appStep 2: Create Migration and Model
Here, we will create migration for "products" table, let's run bellow command and update code.
php artisan make:migration create_products_tabledatabase/migrations/2022_02_21_045036_create_products_table.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('products', function (Blueprint $table) { $table->id(); $table->string('name'); $table->text('detail'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('products'); } };
Next, run create new migration using laravel migration command as bellow:
php artisan migrate
Now we will create Product model by using following command:
app/Models/Prodcut.php<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; /** * Write code on Method * * @return response() */ protected $fillable = [ 'name', 'detail' ]; }Step 3: Create Controller
In this step, we will create a new ProductController; in this file, we will add two method index() and store() for render view and create post with json response.
Let's create ProductController by following command:
php artisan make:controller ProductController
next, let's update the following code to Controller File.
app/Http/Controllers/ProductController.php<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Validator; use App\Models\Product; class ProductController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $products = Product::get(); return view('product', compact('products')); } /** * Write code on Method * * @return response() */ public function store(Request $request) { $validator = Validator::make($request->all(), [ 'name' => 'required', 'detail' => 'required', ]); if ($validator->fails()) { return response()->json([ 'error' => $validator->errors()->all() ]); } Product::create([ 'name' => $request->name, 'detail' => $request->detail, ]); return response()->json(['success' => 'Product created successfully.']); } }Step 4: Create and Add Routes
Furthermore, open routes/web.php file and add the routes to manage GET and POST requests for render view and ajax post request.
routes/web.php<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\ProductController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::controller(ProductController::class)->group(function(){ Route::get('products', 'index'); Route::post('products', 'store')->name('products.store'); });Step 5: Create Blade File
At last step we need to create product.blade.php file and in this file we will display all product and write code for jquery ajax request. So copy bellow and put on that file.
resources/views/product.blade.php<!DOCTYPE html> <html> <head> <title>How to use Ajax Request in Laravel 9? - Mywebtuts.com</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" ></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script> <meta name="csrf-token" content="{{ csrf_token() }}" /> </head> <style type="text/css"> .alert{ padding:15px 0px 0px 0px; } </style> <body> <div class="container"> <div class="card mt-3"> <div class="card-header text-center"> <h3>How to use Ajax Request in Laravel 9? - Mywebtuts.com</h3> </div> <div class="card-body"> <table class="table table-bordered mt-3"> <tr> <th colspan="3"> List Of Products <button type="button" class="btn btn-success float-end btn-sm" data-bs-toggle="modal" data-bs-target="#postModal"> Create Product </button> </th> </tr> <tr> <th>ID</th> <th>Title</th> <th>Body</th> </tr> @foreach($products as $product) <tr> <td>{{ $product->id }}</td> <td>{{ $product->name }}</td> <td>{{ $product->detail }}</td> </tr> @endforeach </table> </div> </div> </div> <!-- Modal --> <div class="modal fade" id="postModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h5 class="modal-title" id="exampleModalLabel">Create Product</h5> <button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button> </div> <div class="modal-body"> <form> <div class="alert alert-danger print-error-msg" style="display:none"> <ul></ul> </div> <div class="mb-3"> <label for="titleID" class="form-label">Name:</label> <input type="text" id="titleID" name="name" class="form-control" placeholder="Name" required=""> </div> <div class="mb-3"> <label for="bodyID" class="form-label">Detail:</label> <textarea name="detail" class="form-control" id="bodyID" placeholder="Detail"></textarea> </div> <div class="mb-3 text-center"> <button class="btn btn-success btn-submit btn-sm">Submit</button> </div> </form> </div> </div> </div> </div> </body> <script type="text/javascript"> $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); $(".btn-submit").click(function(e){ e.preventDefault(); var name = $("#titleID").val(); var detail = $("#bodyID").val(); $.ajax({ type:'POST', url:"{{ route('products.store') }}", data:{name:name, detail:detail}, success:function(data){ if($.isEmptyObject(data.error)){ alert(data.success); location.reload(); }else{ printErrorMsg(data.error); } } }); }); function printErrorMsg (msg) { $(".print-error-msg").find("ul").html(''); $(".print-error-msg").css('display','block'); $.each( msg, function( key, value ) { $(".print-error-msg").find("ul").append('<li>'+value+'</li>'); }); } </script> </html>Run Laravel App:
All steps have been done, now you have to type the given command and hit enter to run the laravel app:
php artisan serve
Now, you have to open web browser, type the given URL and view the app output:
http://localhost:8000/productsOutput:
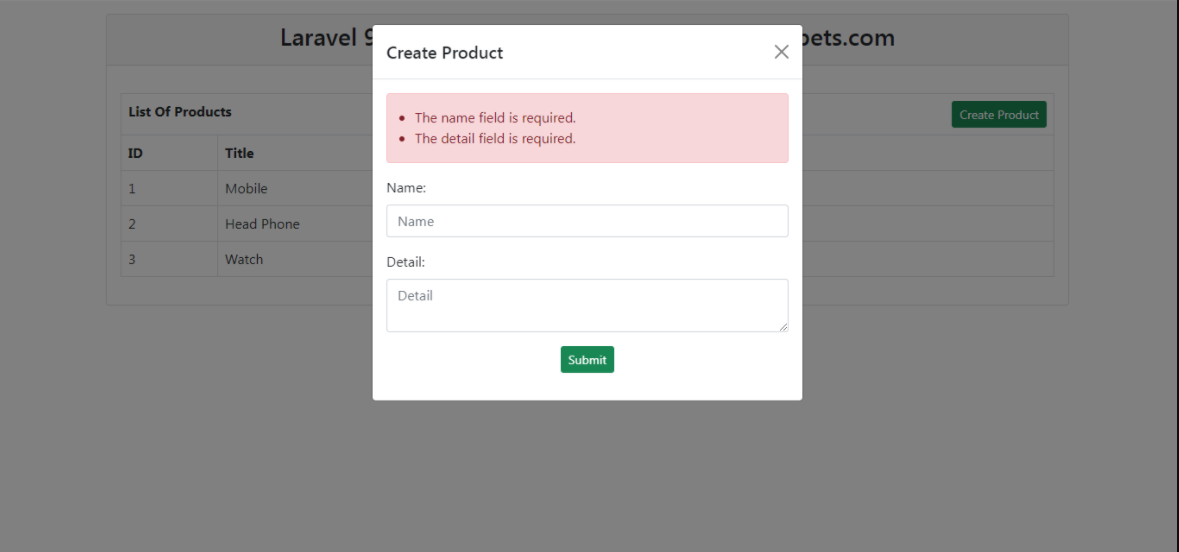
I hope it can help you...