Laravel Inner Join Query Example Tutorial
May 08, 2021 . Admin
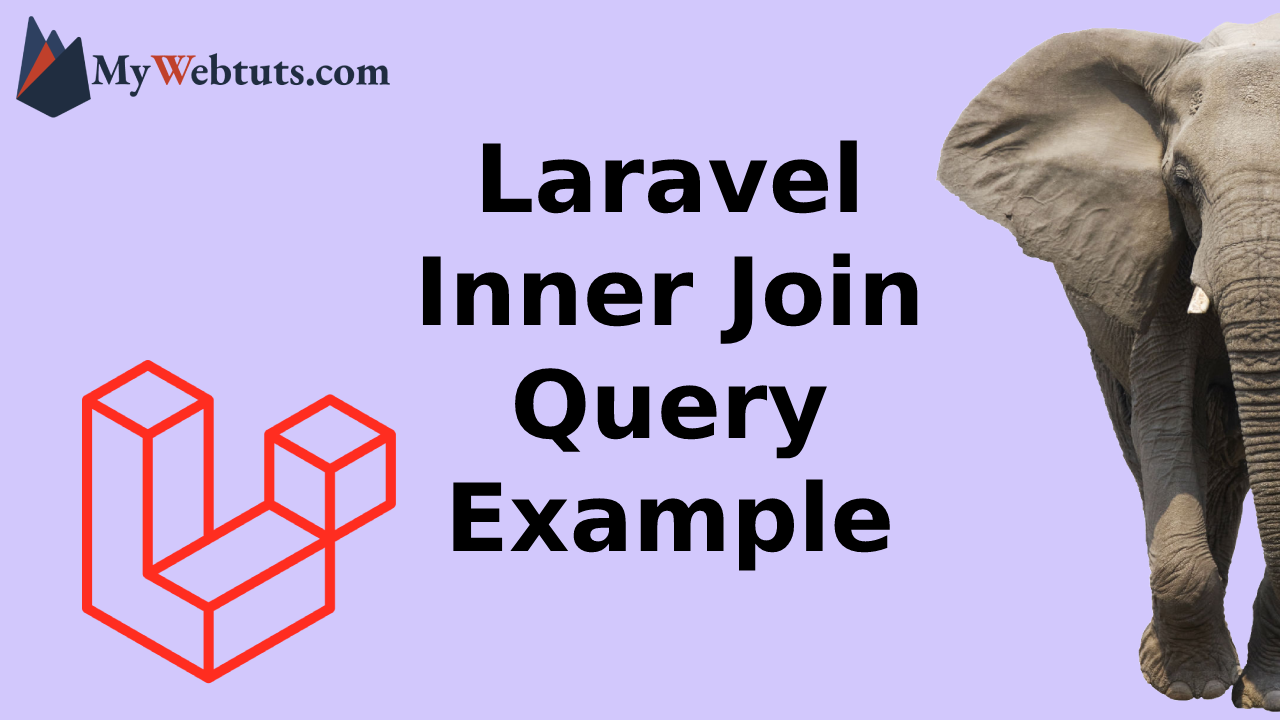
Hello Friends,
Now let's see example of how to use inner join in laravel. This is a short guide on laravel if inner join query. We will use how to use inner join in laravel. Here you will learn how to use inner join in laravel. We will use how to use if inner join in laravel. Let's get started with how to inner join use in laravel.
Here i will give you many example how you can check inner join query in laravel.
Laravel Query:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Admin; class AdminController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $admins = Admin::select( "admins.id", "admins.name", "admins.email", "cities.name as city_name" ) ->join("cities", "cities.id", "=", "admins.city_id") ->get(); dd($admins); } } ?>
Output:
Array ( [0] => Array ( [id] => 1 [name] => John [email] => abc@gmail.com [city_name] => Baroda ) [1] => Array ( [id] => 2 [name] => Delvin [email] => xyz@example.net [city_name] => Bombay ) [2] => Array ( [id] => 3 [name] => Judy [email] => mno@example.org [city_name] => Surat ) )
Now i will give you best way to use inner join and get city name using Laravel:
Example: Using Relationship:You have to create following models and controller method:
app/Admin.php
<?php namespace App; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Foundation\Auth\Admin as Authenticatable; use Illuminate\Notifications\Notifiable; class Admin extends Authenticatable { use Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast to native types. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; /** * Get the comments for the blog post. */ public function country() { return $this->belongsTo(City::class); } } ?>
app/City.php:
<?php namespace App; use Illuminate\Database\Eloquent\Model; class City extends Model { } ?>
Laravel Query:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Admin; class AdminController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $admins = Admin::select("*") ->with('city') ->take(3) ->get(); foreach ($admins as $key => $value) { echo $value->id; echo $value->name; echo $value->city->name; } dd($admins); } } ?>
It will help you....