Laravel Move Data from One Table to Another Table Example
Feb 08, 2023 . Admin
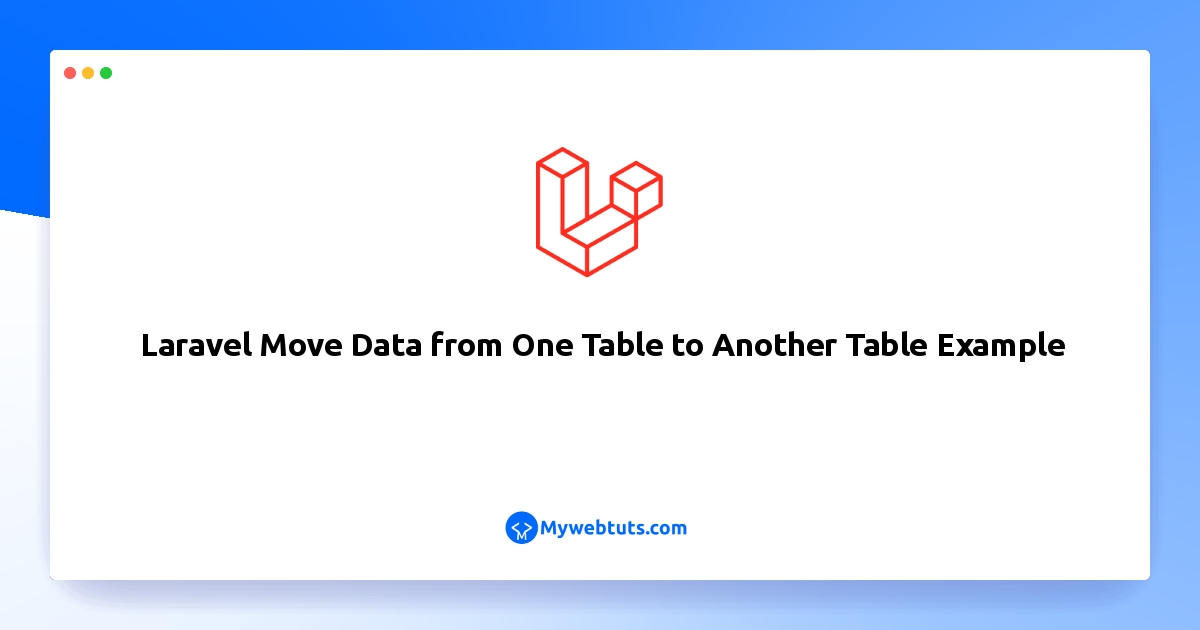
Hi dev,
This tutorial explains how to use Laravel to move data between tables. This article will provide you with a straightforward example of how to migrate data in Laravel from one table to another. I want to explain to you how to move data from one Laravel table to another. You'll discover how to use Laravel to move data from one table to another.
Laravel provides replicate() eloquent method to move records from one table to another table. we will use replicate() with setTable() method to migrate data one table to another. I will give you the following three examples:
Following the code example
Example 1: Laravel Move One Row to Another TableHere, we will use replicate() and setTable() method to move one record to another table.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { $user = User::find(1); $user->replicate() ->setTable('inactive_users') ->save(); } }Example 2: Laravel Move Multiple Row to Another Table
Here, we will use replicate() and setTable() method to move multiple records to another table.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { User::select("*") ->where('last_login','<', now()->subYear()) ->each(function ($user) { $newUser = $user->replicate(); $newUser->setTable('inactive_users'); $newUser->save(); $user->delete(); }); } }Example 3: Laravel Copy Data to Same Table
Here, we will use replicate() and save() method to copy data on same setTable.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Product; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { $product = Product::find(2); $newProduct = $product->replicate()->save(); dd($newProduct); } }
i hope it can help you...