Laravel 8 Image Upload Tutorial Example
May 12, 2021 . Admin

Hi Guys,
Today, I will give you example of laravel 8 image upload example. I’m going to show you about image upload in laravel 8. this example will help you laravel 8 upload image to database. This article goes in detailed on how to upload and exhibit image in laravel 8. Here, Engendering a rudimentary example of laravel 8 image upload with preview.
In this tutorial, we will create two routes one for get method and second one is post method. we engendered simple form with file input. So you have to simple select image and then it will upload in "image" directory of public folder. So you have to simple follow bellow step and get image upload in laravel 8 application.
Here, I will give you full example for laravel 8 image upload as bellow.
Step 1 : Install Laravel 8First of all, we need to create laravel 8 project application using bellow command because of we are going from scratch, So open your terminal OR command prompt and run bellow command:
composer create-project --prefer-dist laravel/laravel blogStep 2: Create Routes
After Install laravel 8 new project we next step, we will add new two routes in web.php file. One route for generate form and another for post method So let's simply create both route as bellow listed:
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\ImageUploadController; // ImageUploadController Route Route::get("image-upload",[ImageUploadController::class,'img_upload'])->name("img.upload"); Route::post("imgstore",[ImageUploadController::class,'imagestore'])->name("img.store");Step 3: Create ImageUploadController
In third step we will have to create new ImageUploadController with simple command i hope already you know how to create controller and here we have to write two method img_upload() and imagestore(). So one method will handle get method another one for post. So let's add code.
app/Http/Controllers/ImageUploadController.php<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class ImageUploadController extends Controller { /** * Display a listing of the resource. * * @return response() */ public function img_upload() { return view('imgUpload'); } /** * Write code on Method * * @return response() */ public function imagestore(Request $request) { $request->validate([ 'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048', ]); $imageName = time().'.'.$request->image->extension(); $request->image->move(public_path('image'),$imageName); // Store $imageName name in DATABASE from HERE return back()->with('success',"image upload successfully")->with('image',$imageName); ; } }Store Image in Storage Folder
$request->image->storeAs('image', $imageName); // storage/app/images/yourfile.pngStore Image in Public Folder
$request->image->move(public_path('image'), $imageName); // public/images/yourfile.pngStore Image in S3
$request->image->storeAs('image', $imageName, 's3');Step 4: Create Blade File
Now,We are going at last step we need to create imgUpload.blade.php file and in this file we will create form with file input button. So copy bellow and put on that file.
resources/views/imgUpload.blade.php<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>laravel 8 image upload example - MyWebtuts.com</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css"> </head> <body class="bg-light"> <div class="container mt-5 border-dark"> <div class="row"> <div class="col-md-12"> <div class="card"> <div class="card-header bg-dark text-white"> <h3>laravel 8 image upload example - MyWebtuts.com</h3> </div> <div class="card-body"> @if($message = Session::get('success')) <div class="alert alert-success alert-block"> <button type="button" class="close" data-dismiss="alert">×</button> <strong>{{ $message }}</strong> </div> <img src="image/{{ Session::get('image') }}"> @endif @if(count($errors) > 0) <div class="alert alert-danger"> <strong>Whoops!</strong> There were some problems with your input. <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form action="{{ route('img.store') }}" method="POST" enctype="multipart/form-data"> @csrf <div class="row"> <div class="col-md-6"> <input type="file" name="image" class="form-control"> </div> <div class="col-md-6"> <button type="submit" class="btn btn-success">Upload</button> </div> </div> </form> </div> </div> </div> </div> </div> </body> </html>Output
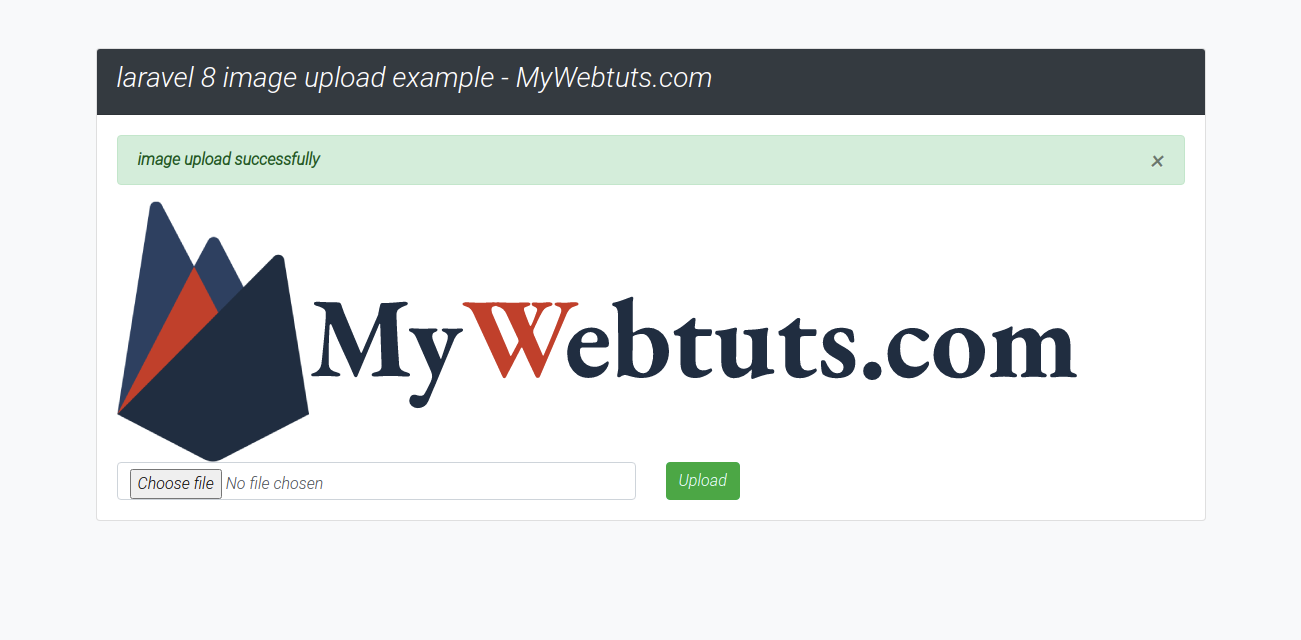
Now you can run and check it.
I Hope It will help you..