Laravel Update All Rows Database Records Example
Dec 27, 2022 . Admin
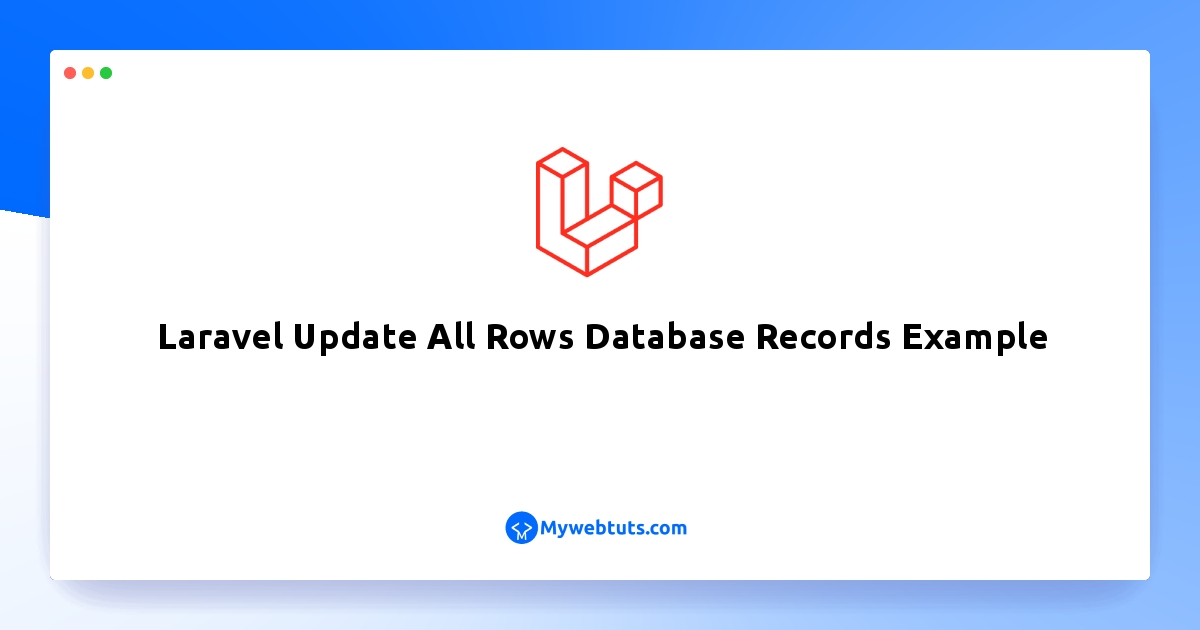
Hello Friends,
This post includes a demonstration of how to update multiple rows in Laravel Eloquent. How to update multiple rows in Laravel was explained in detail. We will help you by giving you a Laravel update multiple records by id example. You'll discover how to use an array in Laravel to update several rows.
Multiple rows in a table can be updated using the where(), update(), and whereIn() and update() methods of Eloquent. I provided three simple examples of updating many products in Laravel Eloquent. Let's examine each of the cases in turn:
Now let's start.
Install LaravelThis is optional; however, if you have not created the laravel app, then you may go ahead and execute the below command:
composer create-project laravel/laravel example-appExample 1: App\Http\Controllers\ProductController
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Product; class ProductController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { Product::where("type", 1) ->update(["color" => "red"]); dd("Products updated successfully."); } }Example 2: App\Http\Controllers\ProductController
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Product; class ProductController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { $ids = [34, 56, 100, 104]; Product::whereIn("id", $ids) ->update([ 'color' => 'blue', 'size' => 'XL', 'price' => 200 ]); dd("Products updated successfully."); } }Example 3: App\Http\Controllers\ProductController
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Product; class ProductController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { $ids = [34, 56, 100, 104]; Product::whereIn("id", $ids) ->update($request->all()); dd("Products updated successfully."); } }
I hope it can help you...