Laravel SMS Notification with Vonage API Tutorial
Dec 19, 2022 . Admin
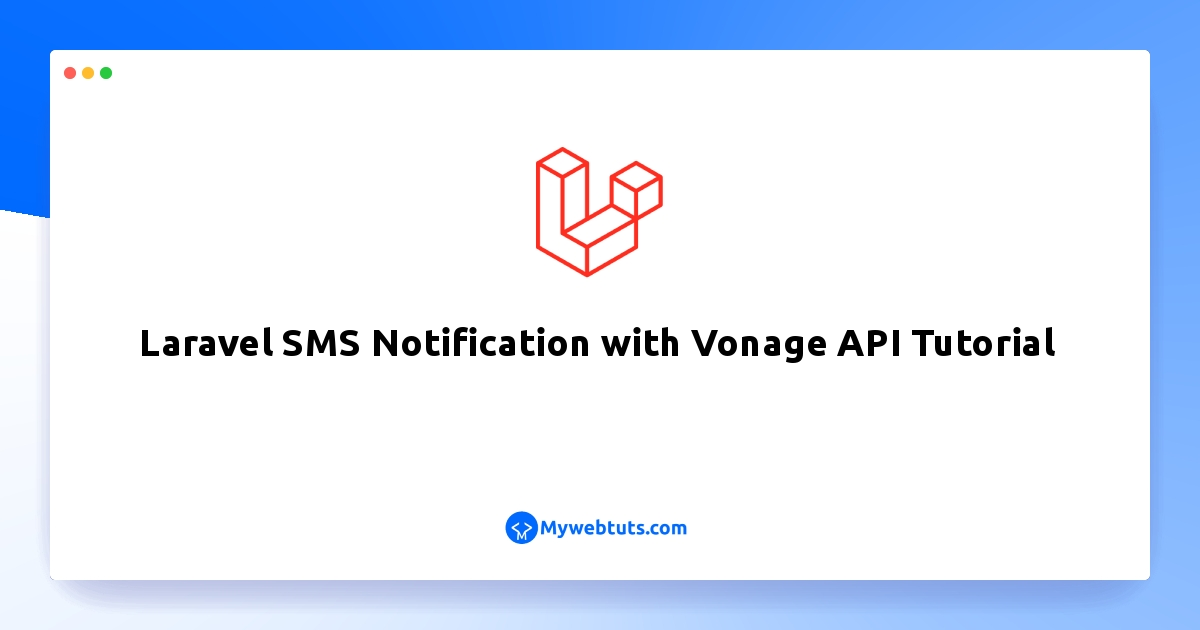
Hello Friends,
I'll explain how to use the Vonage API to build Laravel 9 SMS notification in this tutorial (formerly known as Nexmo). We occasionally need to develop a notice that sends an SMS to your user transaction directly.
We will choose Vonage as our SMS provider because Laravel and the Vonage API work together very well. So please register here if you don't already have an account.
Now let's start.
Step 1: Install LaravelThis is optional; however, if you have not created the laravel app, then you may go ahead and execute the below command:
composer create-project laravel/laravel example-appStep 2: Database Configuration .env
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=your_database_name_here DB_USERNAME=your_database_username_here DB_PASSWORD=your_database_password_hereStep 3: Migration Setup
php artisan notifications:table
We must add this information from your user migration since we are using the phone number column from the user table. See the example code below.
database/migrations/create_users_table.php<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateUsersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('name')->nullable(); $table->string('email')->unique(); $table->string('phone_number')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->softDeletes(); $table->rememberToken(); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('users'); } }
Then once done. Kindly run the following command:
php artisan migrate
Then once done let's create a seeder for our user. Run the following command:
php artisan make:seeder CreateUserSeederdatabase/seeders/UserSeeder
<?php namespace Database\Seeders; use App\Models\User; use Illuminate\Database\Seeder; class CreateUserSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { User::create([ 'name' => 'Juan', 'email' => 'email@gmail.com', 'phone_number' => '63926*******', 'password' => bcrypt('password') ]); } }
Then run the following command:
php artisan db:seed --class=CreateUserSeederStep 4: Install Package & Connect Vonage API
Installing Vonage channel notification through composer is required in order to use Vonage for SMS notifications:
composer require laravel/nexmo-notification-channel
Once installed let's connect Vonage API to our Laravel App. Login to your Vonage account and click "Getting started".
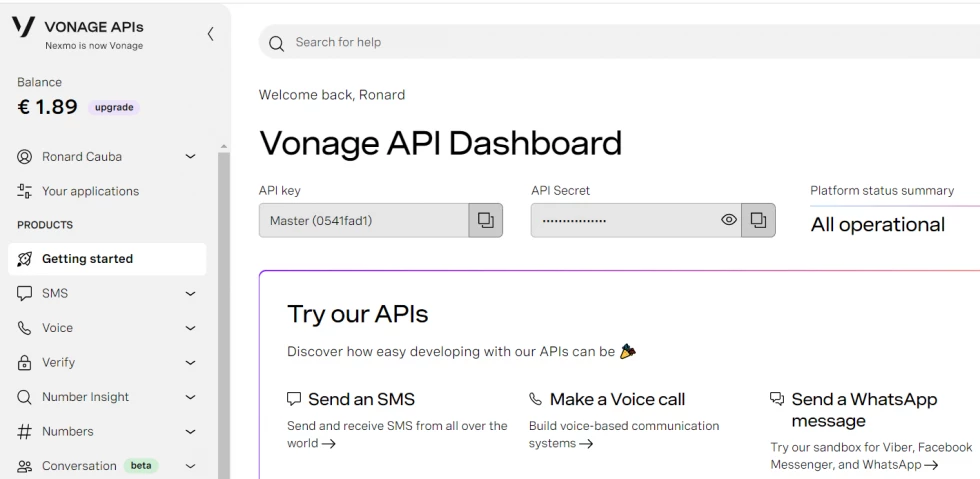
Then add the credentials to your .ENV file.
.envNEXMO_KEY=key_here NEXMO_SECRET=secret_here
Next, we will add sms_from it to our config/services.php file. The sms_from is the phone number to use for sending messages and will be sent from.
config/services.php'nexmo' => [ 'sms_from' => 'Vonage SMS API HERE', ],Step 5: Create Laravel SMS Notification
Now, let's generate our Laravel SMS notification class example we will name this as SMSNotification. Run the following command to do this.
php artisan make:notification SMSNotificationApp/Notifications/EmailNotification.php
<?php namespace App\Notifications; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Notification; use Illuminate\Notifications\Messages\NexmoMessage; class SMSNotification extends Notification { use Queueable; /** * @var array $project */ protected $project; /** * Create a new notification instance. * * @return void */ public function __construct($project) { $this->project = $project; } /** * Get the notification's delivery channels. * * @param mixed $notifiable * @return array */ public function via($notifiable) { return ['mail','database','nexmo']; } /** * Get the mail representation of the notification. * * @param mixed $notifiable * @return \Illuminate\Notifications\Messages\MailMessage */ public function toMail($notifiable) { return (new MailMessage) ->greeting($this->project['greeting']) ->line($this->project['body']) ->action($this->project['actionText'], $this->project['actionURL']) ->line($this->project['thanks']); } /** * Get the array representation of the notification. * * @param mixed $notifiable * @return array */ public function toDatabase($notifiable) { return [ 'project_id' => $this->project['id'] ]; } /** * Get the Nexmo / SMS representation of the notification. * * @param mixed $notifiable * @return NexmoMessage */ public function toNexmo($notifiable) { return (new NexmoMessage) ->content($this->project['greeting'] . ' ' . $this->project['body']); } /** * Get the array representation of the notification. * * @param mixed $notifiable * @return array */ public function toArray($notifiable) { return [ // ]; } }Step 6: Setting up Routes routes/web.php
use Illuminate\Support\Facades\Route; use App\Http\Controllers\HomeController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('/', function () { return view('welcome'); }); Route::get('/send',[HomeController::class,'send'])->name('home.send');Step 7: Setting Up Controller App/Http/Controllers/HomeController
<?php namespace App\Http\Controllers; use Notification; use App\Models\User; use Illuminate\Http\Request; use App\Notifications\SMSNotification; class HomeController extends Controller { public function send() { $user = User::first(); $project = [ 'greeting' => 'Hi '.$user->name.',', 'body' => 'This is the project assigned to you.', 'thanks' => 'Thank you this is from codeanddeploy.com', 'actionText' => 'View Project', 'actionURL' => url('/'), 'id' => 57 ]; Notification::send($user, new SMSNotification($project)); dd('Notification sent!'); } }App\Models\User
<?php use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Laravel\Sanctum\HasApiTokens; class User extends Authenticatable { use HasApiTokens, HasFactory, Notifiable; /** * The attributes that are mass assignable. * * @var string[] */ protected $fillable = [ 'name', 'email', 'password', 'phone_number', ]; /** * The attributes that should be hidden for serialization. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; public function routeNotificationForNexmo($notification) { return $this->phone_number; } }
We can now test our Laravel-based SMS notification.
You can test it now by running the serve command:
php artisan serve
Then run the URL below to your browser to send an SMS notifications to your user.
http://127.0.0.1:8000/sendOutput
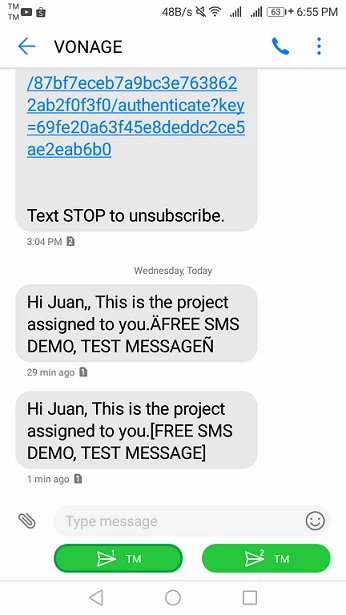
I hope it can help you...