Laravel 10 Model Events Example Tutorial
Apr 12, 2023 . Admin
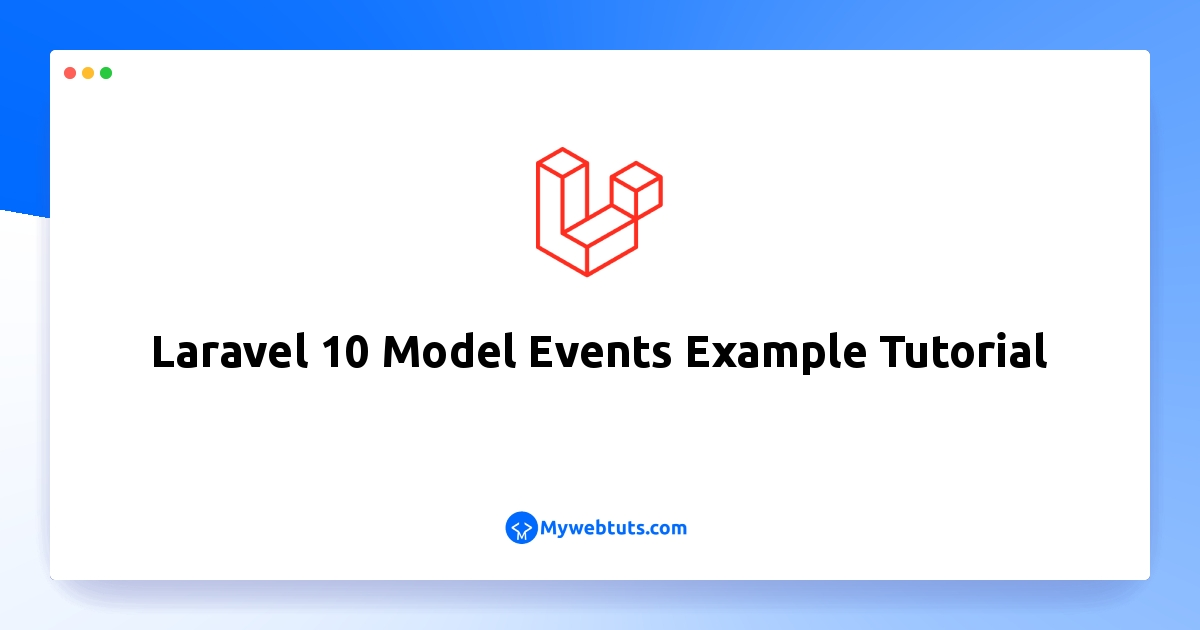
Hi dev,
I'll be explaining how to use the Laravel 10 model events tutorial today. We'll look at the Laravel 10 Model events tutorial concept in this article. The post provides you with sensitive details about Laravel model developments. In Laravel 10, the model-creating event is simple and convenient to use. I'll demonstrate how to use the model to create events in Laravel 10 in this example.
If you are looking for an article about model events in laravel, then you are at a good place to learn about model events and their role to use. Step-by-step guide for model events, you are going to learn.
So let's start with the following example:
creating:
created: Call After Created Record.
updating: Call Before Update Record.
updated: Class After Updated Record.
deleting: Call Before Delete Record.
deleted: Call After Deleted Record.
retrieved: Call Retrieve Data from Database.
saving: Call Before Creating or Updating Record.
saved: Call After Created or Updated Record.
restoring: Call Before Restore Record.
restored: Call After Restore Record.
replicating: Call on replicate Record.
Sometimes, we need to add a unique number or create a slug from the title before creating a record you need to update these fields at a time we can use those times of events. the same thing when you update records then also you have to update the slug and might when removing records then also you have to delete child records. so the event is very helpful and I will say you must have used it when you need this type of situation.
I will explain a few important events with examples so you will understand how it works and how you can use them.
In this example, I will write down creating, created, updating, updated, and deleted events and I will show you a one-by-one example with output in the log file so you can easily understand, how model events work.
Download LaravelLet us begin the tutorial by installing a new laravel application. if you have already created the project, then skip the following step.
composer create-project laravel/laravel example-appCreate Post Model with events
Here, we will create a post-model with events. so let's create and put bellow code:
php artisan make:model Postapp/Models/Post.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; use Log; use Str; class Post extends Model { use HasFactory; protected $fillable = [ 'title', 'slug', 'body' ]; /** * Write code on Method * * @return response() */ public static function boot() { parent::boot(); /** * Write code on Method * * @return response() */ static::creating(function($item) { Log::info('Creating event call: '.$item); $item->slug = Str::slug($item->title); }); /** * Write code on Method * * @return response() */ static::created(function($item) { /* Write Logic Here */ Log::info('Created event call: '.$item); }); /** * Write code on Method * * @return response() */ static::updating(function($item) { Log::info('Updating event call: '.$item); $item->slug = Str::slug($item->name); }); /** * Write code on Method * * @return response() */ static::updated(function($item) { /* Write Logic Here */ Log::info('Updated event call: '.$item); }); /** * Write code on Method * * @return response() */ static::deleted(function($item) { Log::info('Deleted event call: '.$item); }); } }
Now here, we will simply call one by one model method, and let's see the output:
Create Record: Creating and Created Eventphp artisan make:controller PostControllerapp/Http/Controllers/PostController.php
<?php namespace App\Http\Controllers; use App\Models\Post; use Illuminate\Http\Request; class PostController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { Post::create([ 'title' => 'Laravel', 'body' => 'This is a PHP Framework.' ]); dd('done'); } }Output Log File:
[2020-10-20 14:37:26] local.INFO: Creating event call: {"title":"Laravel","body":"This is a PHP Framework."} [2020-10-20 14:37:26] local.INFO: Created event call: {"title":"Laravel","body":"This is a PHP Framework.","slug":"laravel","updated_at":"2022-03-12T14:37:26.000000Z","created_at":"2022-03-12T14:37:26.000000Z","id":1}Update Record: Updating and Updated Event app/Http/Controllers/PostController.php
<?php namespace App\Http\Controllers; use App\Models\Post; use Illuminate\Http\Request; class PostController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { Post::find(1)->update([ 'title' => 'Laravel updated', 'body' => 'This is a PHP Framework updated' ]); dd('done'); } }Output Log File:
[2020-10-20 14:39:04] local.INFO: Updating event call: {"id":1,"title":"Laravel updated","body":"This is a PHP Framework updated","created_at":"2022-03-20T14:45:26.000000Z","updated_at":"2022-03-20T14:37:26.000000Z","slug":"silver"} [2020-10-20 14:39:04] local.INFO: Updated event call: {"id":1,"title":"silver updated","body":"This is a PHP Framework updated","created_at":"2022-03-20T14:37:26.000000Z","updated_at":"2022-03-20T14:39:04.000000Z","slug":"silver-updated"}Delete Record: Deleted Event app/Http/Controllers/PostController.php
<?php namespace App\Http\Controllers; use App\Models\Post; use Illuminate\Http\Request; class PostController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { Post::find(1)->delete(); dd('done'); } }Output Log File:
[2020-10-21 03:14:45] local.INFO: Deleted event call: {"id":1,"title":"laravel updated","body":"This is a PHP Framework updated","created_at":"2020-10-20T14:37:26.000000Z","updated_at":"2020-10-20T14:39:04.000000Z","slug":"silver-updated"
Now you can check from your end.
I hope it can help you...