Laravel 8 Image Upload Tutorial
Apr 05, 2021 . Admin
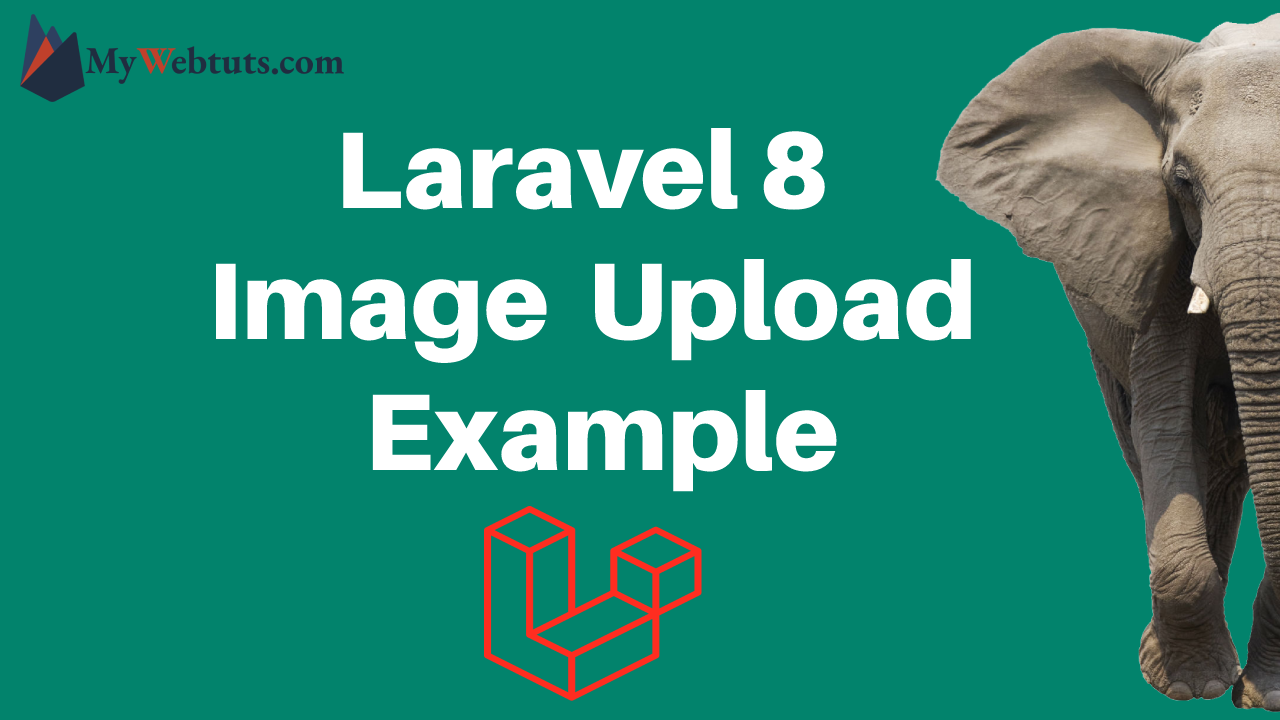
Hi Guys,
Today,I will learn you how to create Image Upload in laravel 8. We will show example of image upload in laravel 8. you can easyliy create Image Upload in laravel 8 I am going to show you about image upload in laravel 8. this example will help you laravel 8 upload image to database. This article goes in detailed on how to upload and display image in laravel 8. Here, Creating a basic example of laravel 8 image upload with preview.
I created simple form with file input. So you have to simple select image and then it will upload in "images" directory of public folder. So you have to simple follow bellow step and get image upload in laravel 8 application.
Here, I will give you full example for simply Image Upload using Laravel 8 as bellow.
Step 1 : Install Laravel 8 Applicationwe are going from scratch, So we require to get fresh Laravel application using bellow command, So open your terminal OR command prompt and run bellow command:
composer create-project --prefer-dist laravel/laravel blogImageUpload cd blogImageUploadStep 2: Create Routes
In next step, we will add new two routes in web.php file. One route for generate form and another for post method So let's simply create both route as bellow listed:
Route::get('/upload-image', [ImageUploadController::class, 'index'])->name('image.upload.index'); Route::post('/upload-image/store', [ImageUploadController::class, 'store'])->name('image.upload.store')Step 3: Create Controller
here this step now we should create new controller as ImageUploadController,So run bellow command for generate new controller
php artisan make:controller ImageUploadController
At last step we need to create imageUpload.blade.php file and in this file we will create form with file input button. So copy bellow and put on that file.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class ImageUploadController extends Controller { /** * Write code on Method * * @return response() */ public function index() { return view('imageUpload'); } /** * Write code on Method * * @return response() */ public function store(Request $request) { $request->validate([ 'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:1024', ]); $imageName = time().'.'.$request->image->extension(); $request->image->move(public_path('images'), $imageName); / store image in database from here / return redirect()->back()->with('success','Image uploaded successfully.')->with('image',$imageName); } }Store Image in Storage Folder
$request->image->storeAs('images', $imageName); // storage/app/images/file.pngStore Image in Public Folder
$request->image->move(public_path('images'), $imageName); // public/images/file.pngStore Image in S3
$request->image->storeAs('images', $imageName, 's3');Step 4: Create Blade File
At last step we need to create imageUpload.blade.php file and in this file we will create form with file input button. So copy bellow and put on that file. resources/views/imageUpload.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel 8 Multiple File Upload - Mywebtuts.com</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.css" integrity="sha256-NuCn4IvuZXdBaFKJOAcsU2Q3ZpwbdFisd5dux4jkQ5w=" crossorigin="anonymous" /> <link rel="stylesheet" type="text/css" href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> <script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="sha384-UO2eT0CpHqdSJQ6hJty5KVphtPhzWj9WO1clHTMGa3JDZwrnQq4sF86dIHNDz0W1" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" integrity="sha384-JjSmVgyd0p3pXB1rRibZUAYoIIy6OrQ6VrjIEaFf/nJGzIxFDsf4x0xIM+B07jRM" crossorigin="anonymous"></script> </head> <body> <div class="container"> <div class="row"> <div class="col-md-6 offset-3 mt-5"> <div class="card mt-5"> <div class="card-header text-center bg-primary"> <h2 class="text-white"> <strong>Multiple File Upload</strong></h2> </div> <div class="card-body"> @if ($message = Session::get('success')) <div class="alert alert-success alert-block"> <button type="button" class="close" data-dismiss="alert">×</button> <strong>{{ $message }}</strong> </div> @endif @if (count($errors) > 0) <ul class="alert alert-danger pl-5"> @foreach($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> @endif <form method="post" action="{{ route('multiple.file.upload.store') }}" enctype="multipart/form-data"> @csrf <div class="input-group file-div control-group lst increment" > <input type="file" name="files[]" class="myfrm form-control"> <div class="input-group-btn"> <button class="btn btn-success add-btn" type="button"><i class="fldemo fa fa-plus"></i></button> </div> </div> <div class="clone hide"> <div class="file-div control-group lst input-group" style="margin-top:10px"> <input type="file" name="files[]" class="myfrm form-control"> <div class="input-group-btn"> <button class="btn btn-danger" type="button"><i class="fldemo fa fa-close"></i></button> </div> </div> </div> <div class="row"> <div class="col-md-12 text-center mt-4"> <button type="submit" class="btn btn-success rounded-0"><i class="fa fa-save"></i> Save</button> </div> </div> </form> </div> </div> </div> </div> </div> <script type="text/javascript"> $(document).ready(function() { $(".add-btn").click(function(){ var lsthmtl = $(".clone").html(); $(".increment").after(lsthmtl); }); $("body").on("click",".btn-danger",function(){ $(this).parents(".file-div").remove(); }); }); </script> </body> </html>
Now we are ready to run our custom validation rules example so run bellow command for quick run:
php artisan serveNow you can open bellow URL on your browser:
http://localhost:8000/upload-image
It will help you...