Laravel Select2 Dynamic Autocomplete Search Example
May 27, 2021 . Admin
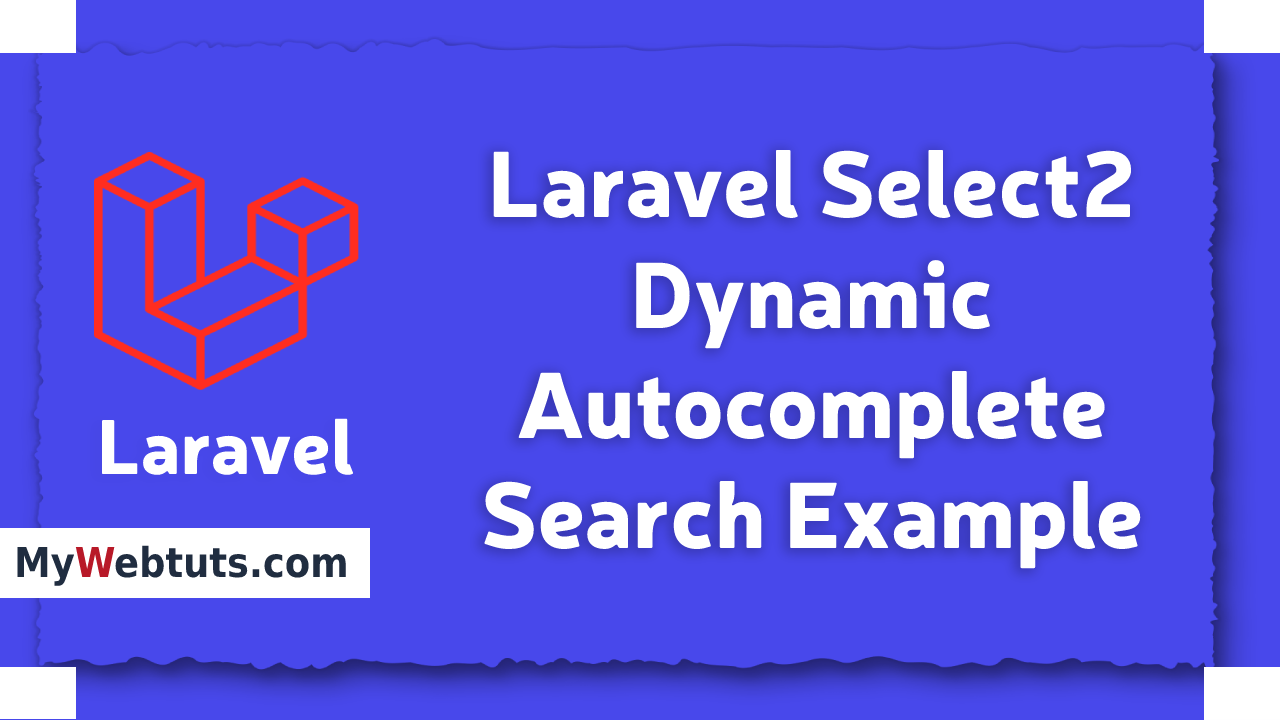
Hey Artisan,
Today, i will explain you how to select2 dynamic autocomplete search using laravel I will create auto complete using Select2 and Ajax in laravel. we will show auto complete using select2.js in laravel. you can easily create auto complete using select2 and ajax in laravel.
I will give you full example of select2 ajax in laravel you can check that code and follow my all step.
Step 1 : Install Laravel 8 ApplicationNow, first step we are going from scratch, So we require to get fresh Laravel 8 application using bellow command, So open your terminal OR command prompt and run bellow command:
composer create-project --prefer-dist laravel/laravel blog
In this step, we need to make database configuration, you have to add following details on your .env file.
- Database Username
- Database Password
- Database name
In .env file also available host and port details, you can configure all details as in your system, So you can put like as bellow:
.envDB_HOST=localhost DB_DATABASE=homestead DB_USERNAME=homestead DB_PASSWORD=secretStep 2: Create students Table and Model
In second step we have to engender migration for students table using Laravel 8 php artisan command, so first fire bellow command:
php artisan make:model Student -m
After this command you have to put bellow code in your migration file for engender students table.
Path : /database/migrations/2021_05_27_100722_create_students_table.php<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateStudentsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('students', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email'); $table->integer('password'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('students'); } }
Now we require to run migration be bellow command:
php artisan migrate
After you have to put bellow code in your model file for create students table.
Path : /App/Models/Student.php<?php namespace App; use Illuminate\Database\Eloquent\Model; class Student extends Model { /** * Write code on Method * * @return response() */ protected $fillable = [ 'name', 'email','password', ]; }Step 3: Create Route
now, we require to integrate resource route for select2 crud operations in laravel application. so open your "routes/web.php" file and add following route.
Path : /routes/web.php<?php use App\Http\Controllers\AutocompleteController; use Illuminate\Support\Facades\Route; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('select2', [AutocompleteController::class, 'index'])->name('select2'); Route::get('/select2-autocomplete', [AutocompleteController::class, 'ajaxdata'])->name('select2-autocomplete');Step 4: Create Controller
Now,in this step we should create new controller as AutocompleteController. So run bellow command and create new controller.
php artisan make:controller AutocompleteController
Now ,in this controller engender two method :
- index()
- ajaxdata()
So, let's copy bellow code and put on AutocompleteController.php file.
Path : app/Http/Controllers/AutocompleteController.php<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; class AutocompleteController extends Controller { /** * Write code on Method * * @return response() */ public function index() { return view('index'); } /** * Write code on Method * * @return response() */ public function ajaxdata(Request $request) { $data = []; if ($request->has('q')) { $search = $request->q; $data = Student::select("id","name")->where('name','LIKE',"%$search%")->get(); } return response()->json($data); } }Step 5:Create Blade File
In the last step we have to engender index.blade.php files. So finally you have to create following bellow blade file:
here create following file and put bellow code.
Path : /resources/views/index.blade.php<!DOCTYPE html> <html> <head> <title>Laravel - Dynamic autocomplete search using select2 JS Ajax</title> <link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.0.0-alpha1/css/bootstrap.min.css"> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.js"></script> <link href="https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.3/css/select2.min.css" rel="stylesheet" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.3/js/select2.min.js"></script> <style> body{ padding: 50px; background-color: #f7fcff; } </style> </head> <body> <div class="container mt-4"> <div class="row"> <div class="col-md-10 mx-auto"> <div class="card"> <div class="card-header bg-dark text-white text-center"> <h4>Laravel Select2 Dynamic Autocomplete Search - Mywebtuts.com</h4> </div> <div class="card-body"> <div class="row"> <div class="col-md-12"> <select class="itemName form-control" name="itemName"></select> </div> </div> </div> </div> </div> </div> </div> </body> <script type="text/javascript"> $('.itemName').select2({ placeholder: 'Select an item', ajax: { url: '/select2-autocomplete', dataType: 'json', delay: 250, processResults: function (data) { return { results: $.map(data, function (item) { return { text: item.name, id: item.id } }) }; }, cache: true } }); </script> </html>
Now we are ready to run our example so run bellow command for quick run:
php artisan serve
Now you can open bellow URL on your browser:
http://localhost:8000/select2Output
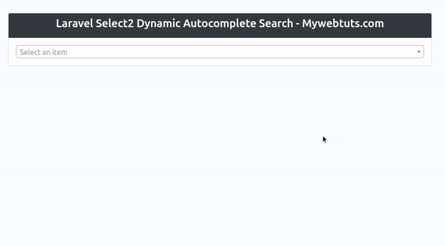