How to Create Enum Column Add Migration In Laravel 9?
Jul 30, 2022 . Admin
This tutorial shows you laravel 9 migration add enum value. you can understand a concept of how to add enum column in laravel 9 migration. We will look at example of enum type in laravel 9 migration. This post will give you simple example of enum type in laravel 9.
Sometime we take column like status and you have specified values for it like pending, active, inactive etc. at that time you can choose enum data type in mysql. but if you want to add enum data type in laravel migration then how you can add enum data type column in laravel.
let's see bellow examples:
Example 1:<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('blogs', function (Blueprint $table) { $table->id(); $table->string('title'); $table->text('body'); $table->enum('status', ['Pending','Active','Inactive']); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('blogs'); } };Example 2: Add Enum Column with Default Value:
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('blogs', function (Blueprint $table) { $table->id(); $table->string('title'); $table->text('body'); $table->enum('status', ['Pending','Active','Inactive'])->default('Pending'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('blogs'); } };Output:
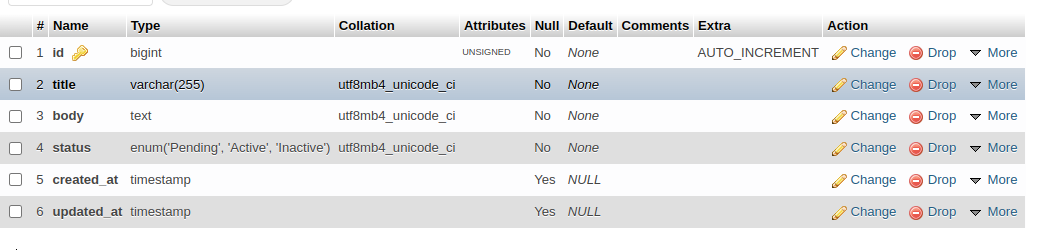
It will help you...