Laravel Migration Change Column Name and Data Type Example
Jan 19, 2023 . Admin
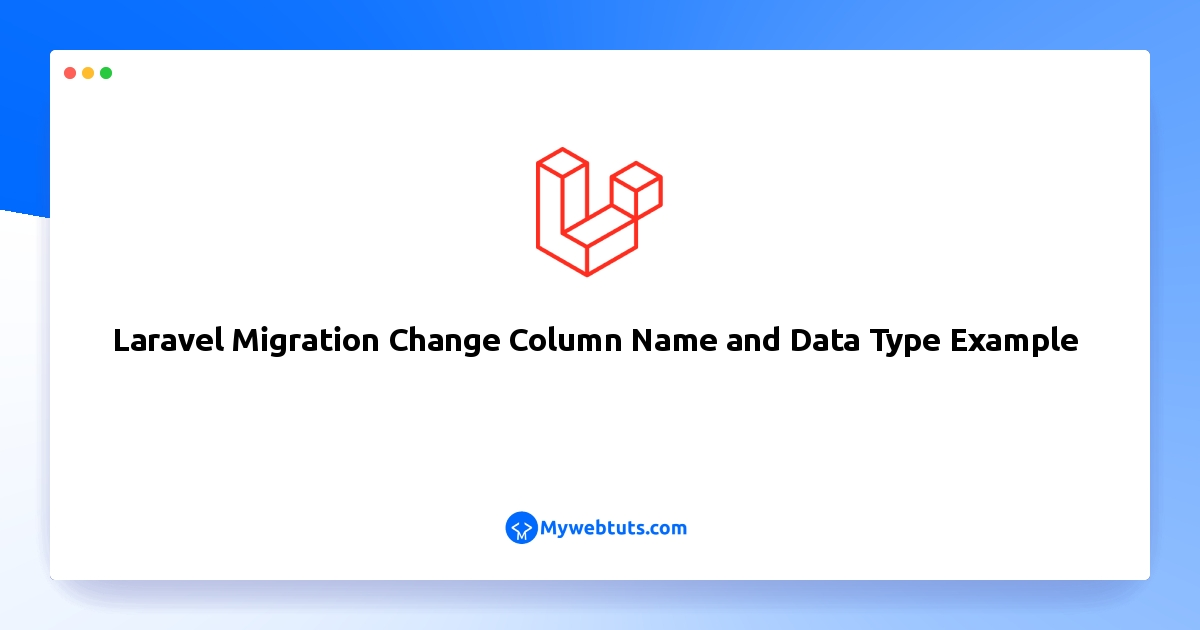
Hi dev
The focus of this post is chanje column name migration in laravel. using this example, you can learn how to change a column's type in a laravel migration. it demonstrates step-by-step how to rename a column's name in a laravel migration. i merely explained how to change the data type in laravel migration. the steps below can be used to change the column names in the laravel migration.
I'll give you two examples of data type changes and column renaming using migration in applications built with Laravel 6, Laravel 7, Laravel 8, and Laravel 9.
Install Composer Package:composer require doctrine/dbal
After successfully install composer package we can change data type and change column name using migration.
Let's see bellow example:
Migration for main table:<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreatePostsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('posts', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('title'); $table->text('body'); $table->boolean('is_publish')->default(0); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('posts'); } }Change Data Type using Migration:
body column text data type to long text data type here
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class ChangePostsTableColumn extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::table('posts', function (Blueprint $table) { $table->longText('body')->change(); }); } /** * Reverse the migrations. * * @return void */ public function down() { } }Rename using Migration:
rename title column to name changed.
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class ChangePostsTableColumn extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::table('posts', function (Blueprint $table) { $table->renameColumn('title', 'name'); }); } /** * Reverse the migrations. * * @return void */ public function down() { } }
I hope it can help you...