React JS Axios DELETE Request Example
Aug 31, 2022 . Admin
Hi Artisan,
In this short guide, we will show you react js axios delete request example. if you want to see an example of react js axios delete example then you are in the right place. If you have a question about react.js axios delete example then I will give a simple example with a solution. I would like to show you react axios delete json example. Let's get started with how to make axios delete call in react js.
In this example, i will show you how to make Axios Delete Request in React JS App. we will install axios using npm command. Then we will use "jsonplaceholder" demo api for get all users and delete post using delete request. we will create simple table with ID, Title and Body and get data from get request and add delete button to remove post.
So, let's see follow the below step to create simple example with axios get request.
Step 1: Create React JS AppIn this step, open your terminal and execute the following command on your terminal to create a new react app:
npx create-react-app react-axios-exampleStep 2: Install Axios npm Package
In this step, we need to install axios npm package to use their functions. let's run below command:
npm install axiosStep 3: Install Bootstrap
In this step, we will install bootstrap npm package to use design css. let's run below command:
npm install bootstrap --saveStep 4: Update App.js Component
Here, we will call two apis for getting posts and another for deleting post using axios request.
src/App.jsimport '../node_modules/bootstrap/dist/css/bootstrap.min.css'; import React from 'react'; import axios from 'axios'; export default class PostList extends React.Component { state = { posts: [] } componentDidMount() { axios.get(`https://jsonplaceholder.typicode.com/posts`) .then(res => { const posts = res.data; this.setState({ posts }); }) } deleteRow(id, e){ axios.delete(`https://jsonplaceholder.typicode.com/posts/${id}`) .then(res => { console.log(res); console.log(res.data); const posts = this.state.posts.filter(item => item.id !== id); this.setState({ posts }); }) } render() { return ( <div class="container"> <h1>React JS Axios Delete Request Example - MyWebtuts.com</h1> <table className="table table-bordered"> <thead> <tr> <th>ID</th> <th>Title</th> <th>Body</th> <th>Action</th> </tr> </thead> <tbody> {this.state.posts.map((post) => ( <tr> <td>{post.id}</td> <td>{post.title}</td> <td>{post.body}</td> <td> <button className="btn btn-danger" onClick={(e) => this.deleteRow(post.id, e)}>Delete</button> </td> </tr> ))} </tbody> </table> </div> ) } }Step 6: Run React JS App
In the last step run your project using bellow command.
npm startOutput
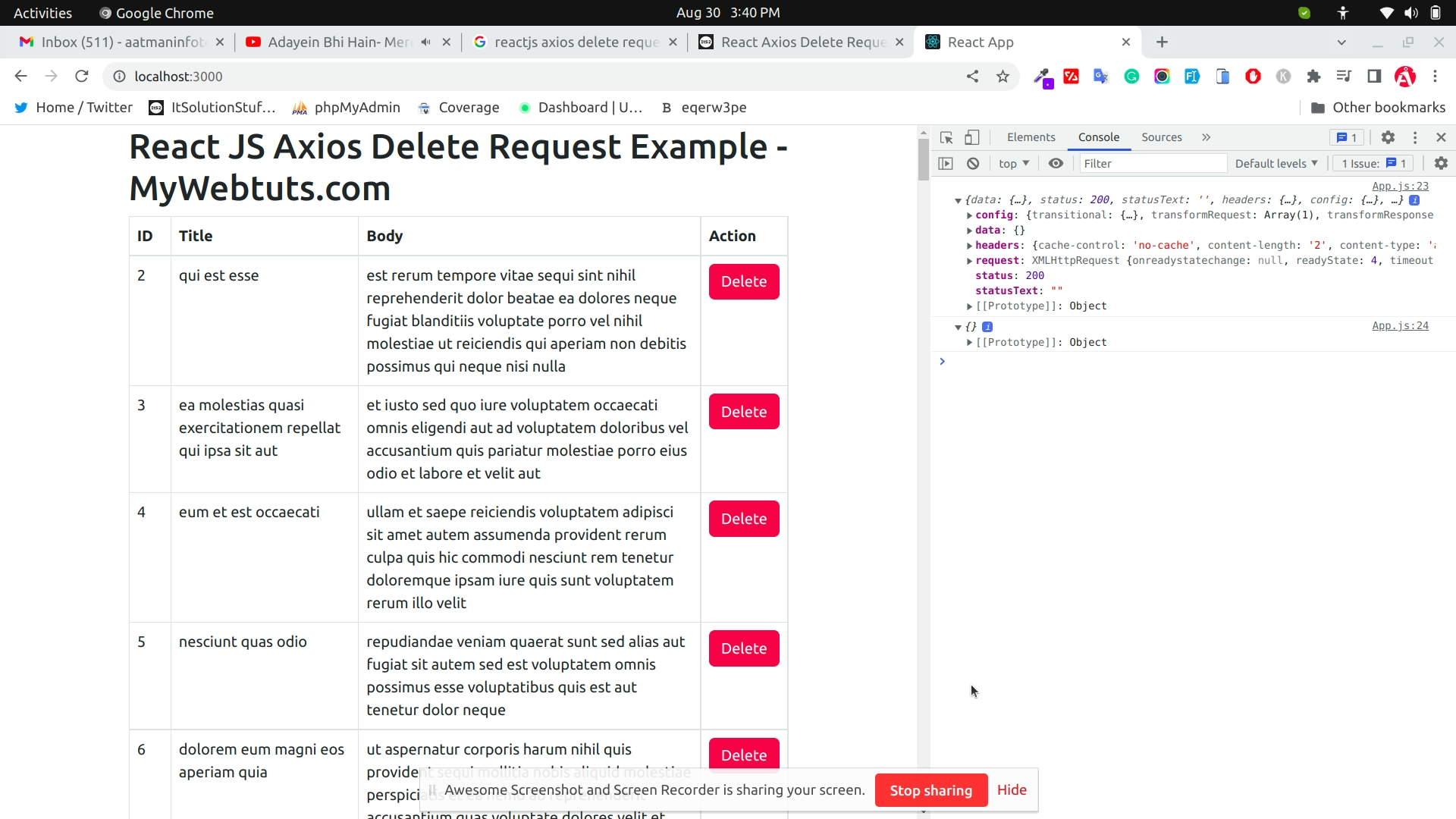
I hope it can help you...