React Native Animated Splash Screen Example
Jul 05, 2022 . Admin
Hi Guys,
In this post, we will learn react native animated splash screen example. I would like to share with you how to implement animated splash screen in react native. This tutorial will give you simple example of animated splash screen example in react native. This article will give you simple example of how to add animated splash screen in react native. Let's see bellow example how to use animated splash screen in react native.
Let's start following example:
Step 1: Download ProjectIn the first step run the following command to create a project.
expo init ExampleAppStep 2: App.js
In this step, You will open the App.js file and put the code.
import React, { useEffect, useState } from 'react'; import { View, StyleSheet, Animated, Text } from 'react-native'; const App = () => { const [isVisible, setisVisible] = useState(true); const width = new Animated.Value(0); const height = new Animated.Value(0); const IMAGE = 'https://tsc-website-production.s3.amazonaws.com/uploads/2018/05/React-Native.png'; useEffect(() => { Animated.timing( width, { toValue: 360, duration: 1200, useNativeDriver: false, }, ).start(); Animated.timing( height, { toValue: 100, duration: 1200, useNativeDriver: false, }, ).start(); }, []); const Hide_Splash_Screen = () => { setisVisible(false); } useEffect(() => { let myTimeout = setTimeout(() => { Hide_Splash_Screen(); }, 3000); return () => clearTimeout(myTimeout); }, []); const Splash_Screen = () => { return ( <View style={styles.container}> <Animated.Image source={{ uri: IMAGE }} style={{ width: width, height: height, position: 'absolute', }} resizeMode='cover' /> </View> ); } return ( <> { (isVisible === true) ? Splash_Screen() : ( <View style={styles.container}> <Text style={styles.title}>Animated Splash Screen Example</Text> </View> ) } </> ); } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#FFF', justifyContent: 'center', alignItems: 'center', }, title: { fontSize:23, fontWeight:'800', }, }); export default App;Run Project
In the last step run your project using the below command.
expo start
You can QR code scan in Expo Go Application on mobile.
Output :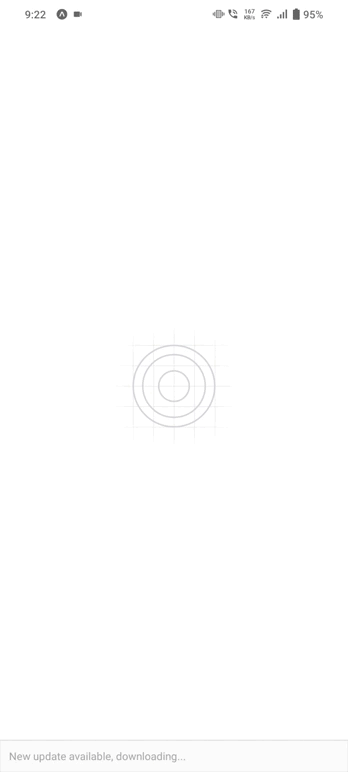
It will help you...