Upload File with Ajax in Laravel 10 Tutorial
Mar 11, 2023 . Admin
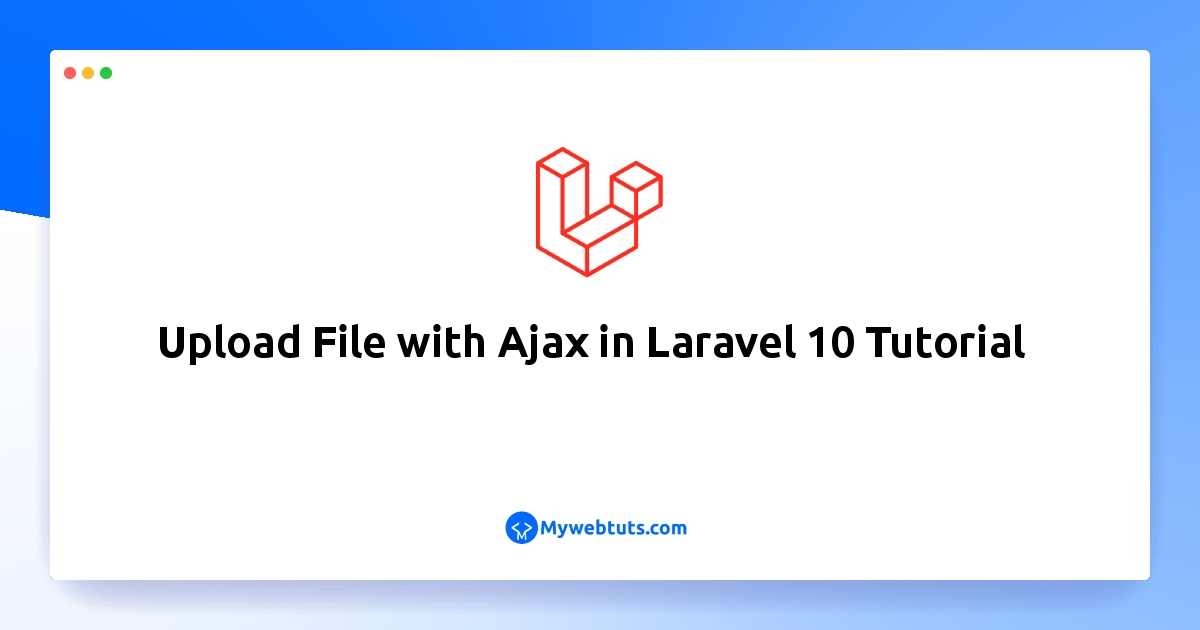
Hi friends,
Today I'll discuss a simple Laravel 10 and ajax example of how to upload a file. This video demonstrates how to utilise Laravel 10's Ajax to upload a file to a folder. In this article, we'll demonstrate how to upload a file in Laravel 10 using an example of ajax and jQuery. This example takes you step-by-step through the Laravel 10 Ajax file upload tutorial. We usually need to save the file data with an ajax call in a Laravel 10 application without reloading the page. In this Laravel 10 Jquery File Upload tutorial, I'll show you how to upload a file using an ajax call. We'll also examine how to validate a file prior to uploading it to a server.
Uploading a file using ajax in Laravel and saving it to the database is quite simple if you are familiar with Laravel form submission using ajax. Specify the path where you want to put the uploaded file when calling the store method.
In Laravel 10, uploading files using an Ajax request is quite simple. Let's begin our tutorial on laravel 10 ajax file upload from scratch.
Step 1: Download LaravelInstalling a fresh Laravel application will kick off the tutorial. If the project has already been created, skip the next step.
composer create-project laravel/laravel example-appStep 2: Add Migration and Model
Here, we will create migration for "files" table, let's run bellow command and update code.
php artisan make:migration create_images_table2022_02_23_054554_create_images_table.php
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('images', function (Blueprint $table) { $table->id(); $table->string('name'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('images'); } };
Next, run create new migration using laravel migration command as bellow:
php artisan migrate
Now we will create File model by using following command:
php artisan make:model Fileapp/Models/File.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class File extends Model { use HasFactory; protected $table = 'images'; protected $fillable = [ 'name' ]; }Step 3: Add Controller
In this step, we will create a new FileUploadController; in this file, we will add two method index() and store() for render view and store files into folder and database logic.
Let's create FileUploadController by following command:
php artisan make:controller FileUploadController
next, let's update the following code to Controller File.
app/Http/Controllers/FileUploadController.php<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\File; class FileUploadController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { return view('fileUpload'); } /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function store(Request $request) { $request->validate([ 'file' => 'required|mimes:doc,docx,pdf,zip,rar|max:2048', ]); $fileName = time().'.'.$request->file->extension(); $request->file->move(public_path('file'), $fileName); File::create(['name' => $fileName]); return response()->json('File uploaded successfully'); } }Store Files in Storage Folder
$file->storeAs('files', $fileName); // storage/app/files/file.pdfStore Files in Public Folder
$file->move(public_path('files'), $fileName); // public/files/file.pdfStore Files in S3
$file->storeAs('files', $fileName, 's3');Step 4: Add Routes
Furthermore, open routes/web.php file and add the routes to manage GET and POST requests for render view and store file logic.
routes/web.php<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\FileUploadController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::controller(FileUploadController::class)->group(function(){ Route::get('file-upload', 'index'); Route::post('file-upload', 'store')->name('file.store'); });Step 5: Add Blade File
At last step we need to create fileUpload.blade.php file and in this file we will create form with file input button and written jquery ajax code. So copy bellow and put on that file.
resources/views/fileUpload.blade.php<!DOCTYPE html> <html> <head> <title>How to Upload File using Ajax in Laravel 10? </title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" integrity="sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ==" crossorigin="anonymous" referrerpolicy="no-referrer"></script> </head> <body> <div class="container"> <div class="panel panel-primary card mt-5"> <div class="panel-heading text-center mt-4"> <h2>How to Upload File using Ajax in Laravel 10?</h2> </div> <div class="panel-body card-body"> <form action="{{ route('file.store') }}" method="POST" id="file-upload" enctype="multipart/form-data"> @csrf <div class="mb-3"> <label class="form-label" for="inputFile">Select File:</label> <input type="file" name="file" id="inputFile" class="form-control"> <span class="text-danger" id="file-input-error"></span> </div> <div class="mb-3"> <button type="submit" class="btn btn-success">Upload</button> </div> </form> </div> </div> </div> </body> <script type="text/javascript"> $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); $('#file-upload').submit(function(e) { e.preventDefault(); let formData = new FormData(this); $('#file-input-error').text(''); $.ajax({ type:'POST', url: "{{ route('file.store') }}", data: formData, contentType: false, processData: false, success: (response) => { if (response) { this.reset(); alert('File has been uploaded successfully'); } }, error: function(response){ $('#file-input-error').text(response.responseJSON.message); } }); }); </script> </html>Run Laravel App: All steps have been done, now you have to type the given command and hit enter to run the laravel app:
php artisan serve
Now, you have to open web browser, type the given URL and view the app output:
http://localhost:8000/file-upload
I hope it can help you...