How to Upload Image with Progress Bar using Ajax and PHP 8?
Jun 23, 2022 . Admin
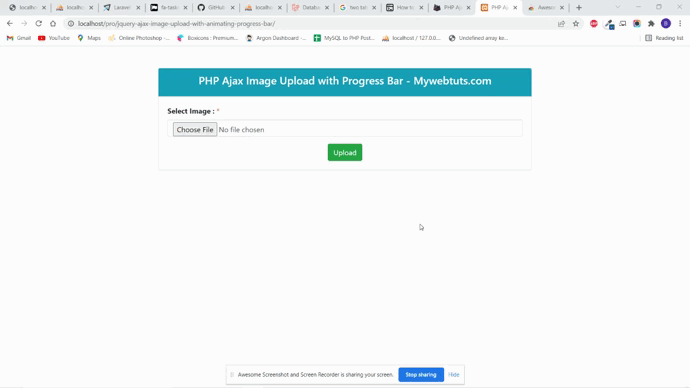
Hello Friends,
This tutorial shows you How to Upload Image with Progress Bar using Ajax and PHP 8?. you'll learn File Upload with Progress Bar using jQuery Ajax and PHP 8. We will use jQuery Ajax Image Upload with Animating Progress Bar in php 8. I explained simply step by step Ajax File Upload with Progress Bar using PHP 8 and JQuery. follow bellow step for Upload Large File with Progress Bar using jQuery Ajax in PHP 8.
This article will give you simple example of PHP 8 Ajax Image Upload with Progress Bar
So, let's see bellow solution:
index.php<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>How to Upload Image with Progress Bar using Ajax and PHP 8?</title> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/css/bootstrap.min.css" integrity="sha384-zCbKRCUGaJDkqS1kPbPd7TveP5iyJE0EjAuZQTgFLD2ylzuqKfdKlfG/eSrtxUkn" crossorigin="anonymous"> <script src="https://code.jquery.com/jquery-3.3.1.min.js" type="text/javascript"></script> <script type="text/javascript" src="jquery.form.min.js"></script> <style type="text/css"> .progress { display: none; position: relative; height: 25px; background-color: #ddd; border-radius: 15px; } .progress-bar { background-color: green; width: 0%; height: 30px; border-radius: 4px; } .percent { position: absolute; display: inline-block; color: #fff; font-weight: bold; top: 87%; left: 50%; margin-top: -9px; margin-left: -20px; } #outputImage { display: none; margin-top: 10px; } #outputImage img { max-width: 300px; } </style> </head> <body> <div class="container mt-5"> <div class="row"> <div class="col-md-12"> <div class="card w-75 m-auto"> <div class="card-header text-center bg-info text-white"> <h4>How to Upload Image with Progress Bar using Ajax and PHP 8? - Mywebtuts.com</h4> </div> <div class="card-body"> <div class='progress' id="progressDivId"> <div class='progress-bar' id='progressBar'></div> <div class='percent' id='percent'>0%</div> </div> <div id='outputImage'></div> <div id='error'></div> <form action="uploadFile.php" id="uploadForm" name="frmupload"method="post" enctype="multipart/form-data"> <div class="form-group"> <label><strong>Select Image : </strong><span class="text-danger"> *</span></label> <input type="file" id="uploadImage" name="uploadImage" class="form-control"> </div> <div class="d-flex justify-content-center"> <input id="submitButton" type="submit" name='btnSubmit' value="Upload" class="btn btn-success"> </div> </form> </div> </div> </div> </div> </div> <script type="text/javascript"> $(document).ready(function () { $('#submitButton').click(function () { $('#uploadForm').ajaxForm({ target: '#outputImage', url: 'uploadFile.php', beforeSubmit: function () { $("#outputImage").hide(); if($("#uploadImage").val() == "") { $("#outputImage").show(); $("#outputImage").html("<div class='alert alert-danger'>Choose a file to upload.</div>"); return false; } $("#progressDivId").css("display", "block"); var percentValue = '0%'; $('#progressBar').width(percentValue); $('#percent').html(percentValue); }, uploadProgress: function (event, position, total, percentComplete) { var percentValue = percentComplete + '%'; $("#progressBar").animate({ width: '' + percentValue + '' }, { duration: 5000, easing: "linear", step: function (x) { percentText = Math.round(x * 100 / percentComplete); $("#percent").text(percentText + "%"); if(percentText == "100") { $("#outputImage").show(); $("#error").html("<div class='alert alert-success mt-2'>Image Successfully Upload.</div>"); } } }); }, error: function (response, status, e) { alert('Oops something went.'); }, complete: function (xhr) { if (xhr.responseText && xhr.responseText != "error") { $("#outputImage").html(xhr.responseText); }else{ $("#outputImage").show(); $("#outputImage").html("<div class='alert alert-danger'>Problem in uploading file.</div>"); $("#progressBar").stop(); } } }); }); }); </script> </body> </html>uploadFile.php
<?php if (isset($_POST['btnSubmit'])) { $uploadfile = $_FILES["uploadImage"]["tmp_name"]; $folderPath = "uploads/"; if (! is_writable($folderPath) || ! is_dir($folderPath)) { echo "error"; exit(); } if (move_uploaded_file($_FILES["uploadImage"]["tmp_name"], $folderPath . $_FILES["uploadImage"]["name"])) { echo '<img src="' . $folderPath . "" . $_FILES["uploadImage"]["name"] . '">'; exit(); } } ?>Output:
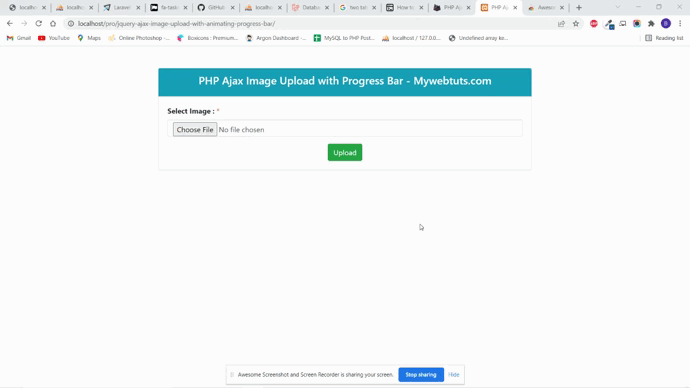
It will help you...