How To Upload Multiple Files in Laravel?
May 27, 2021 . Admin
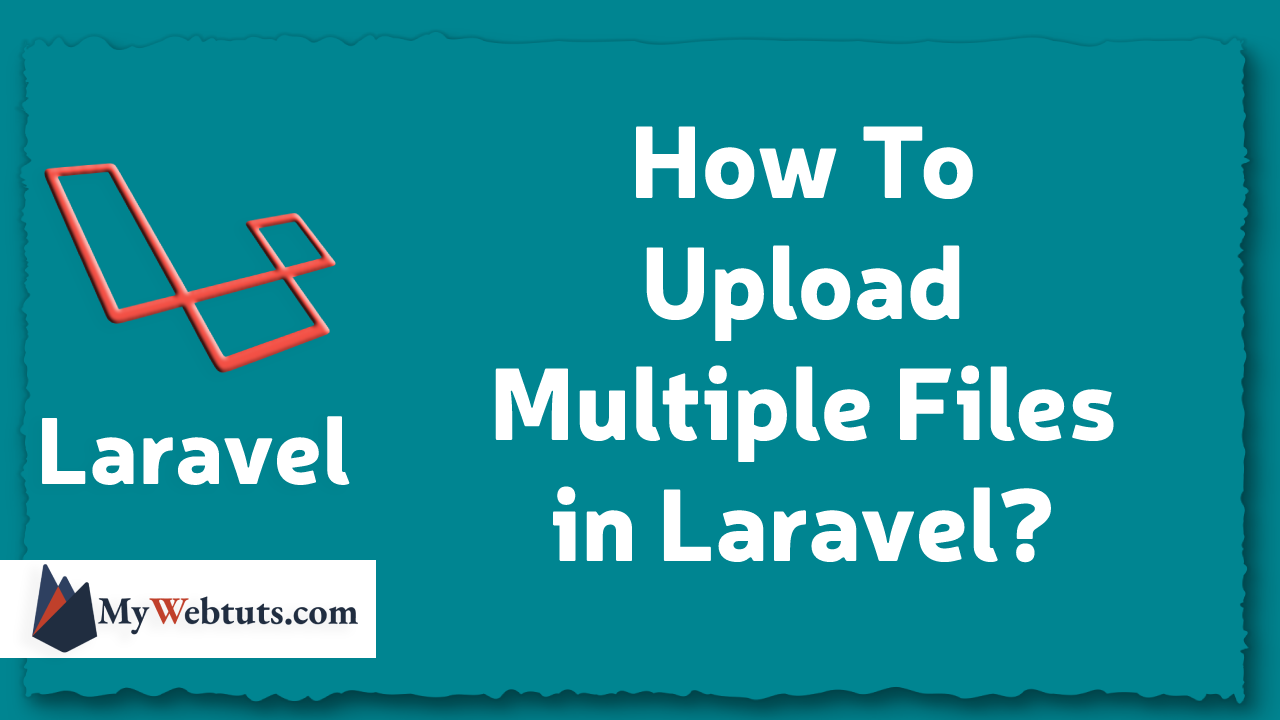
Hello Friends,
Now let's see example of how to upload multiple files in laravel example. This is a short guide on laravel upload multiple files. Here you will learn upload multiple files laravel. We will use upload multiple files in laravel. Let's get started with upload multiple files in laravel.
Here i will give you few step instruction to upload multiple files in laravel.
Step 1: Download LaravelIn the first step, we will download a new simple copy source code of Laravel App project by typing the some following command.
composer create-project --prefer-dist laravel/laravel blogStep 2: Add Migration and Model
Here, we need create database migration for documents table and also we will create model for documents table.
php artisan make:migration create_documents_table
Path: database\migrations\create_documents_table
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateDocumentsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('documents', function (Blueprint $table) { $table->increments('id'); $table->string('files'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('documents'); } }
php artisan migrate
now we will create Document model by using following command:
php artisan make:model Document
<?php namespace App; use Illuminate\Database\Eloquent\Model; class Document extends Model { public $table = 'documents'; protected $fillable = ['files']; }Step 3: Create Routes
In third step, we will create routes for multiple document upload. so create two route with GET and POST route example.
routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\DocumentController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('document/create',[DocumentController::class, 'create']); Route::post('document/store',[DocumentController::class, 'store'])->name('document.store');Step 4: Create Controller
Now we require to add new DocumentController controller for manage route So let's create DocumentController with create and store method. Make sure you need to create "documents" folder in your public directory.
app/Http/Controllers/DocumentController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Document; class DocumentController extends Controller { /** * Write code on Method * * @return response() */ public function create() { return view('create'); } /** * Write code on Method * * @return response() */ public function store(Request $request) { $request->validate([ 'file' => 'required' ]); $files = []; if (!empty($request->file)) { foreach($request->file as $key=>$file) { $filename = time().'-'.$file->getClientOriginalName(); $file->move(public_path('documents'), $filename); $files[] = $filename; } } $files = json_encode($files); // Store $document document in DATABASE from HERE Document::create(['files' => $files]); return back() ->with('success','You have successfully file uplaod.') ->with('files',$filename); } }Step 5: Create Blade File
in this step we need to create create.blade.php file in resources folder. So let's create file:
resources/views/create.blade.php
<html lang="en"> <head> <title>Laravel 8 Multiple File Upload Example</title> <script src="jquery/1.9.1/jquery.js"></script> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous"> <script src="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/js/all.min.js" crossorigin="anonymous"></script> <link rel="stylesheet" href="3.3.6/css/bootstrap.min.css"> </head> <body class="bg-dark"> <div class="container mt-5 lst"> @if (count($errors) > 0) <div class="alert alert-danger"> <strong>Sorry!</strong> There were more problems with your HTML input.<br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif @if(session('success')) <div class="alert alert-success"> {{ session('success') }} </div> @endif <div class="card"> <div class="card-header"> <h3 class="well">Laravel 8 Multiple File upload Example Tutorial</h3> </div> <div class="card-body"> <form method="post" action="{{ route('document.store') }}" enctype="multipart/form-data"> @csrf <div class="row"> <div class="col-md-12"> <div class="form-group"> <label>File:</label> <input type="file" class="form-control" name="file[]" multiple> </div> </div> </div> <div class="row"> <div class="col-md-12"> <button class="btn btn-success btn-block" type="submit">Submit</button> </div> </div> </form> </div> </div> </div> </body> </html>
Now we are ready to run multiple document upload application example with laravel so run bellow command for quick run:
php artisan serve
Now you can open bellow URL on your browser:
localhost:8000/document/create
It will help you....