Laravel 8 Send Email Example
Apr 15, 2021 . Admin
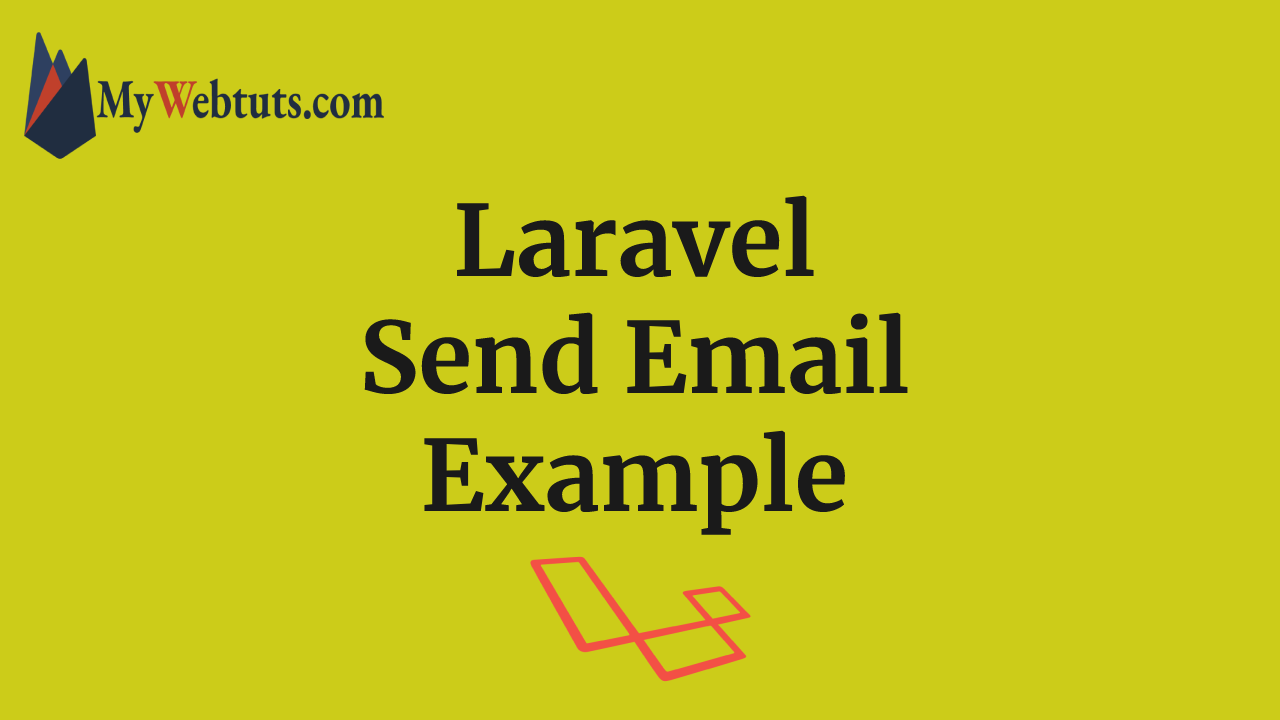
Hello Friends,
Now let's see example of how to send email in laravel example. This is a short guide on laravel send email. Here you will learn how to send laravel email. We will use how to send email in laravel. Let's get started with how to send email in laravel.
Here i will give you few step instruction to send mail in laravel 8.
Step 1: Make ConfigurationIn first step, you have to add send mail configuration with mail driver, mail host, mail port, mail username, mail password so laravel 8 will use those sender details on email. So you can simply add as like following.
.env
MAIL_DRIVER=smtp MAIL_HOST=smtp.gmail.com MAIL_PORT=587 MAIL_USERNAME=mygoogle@gmail.com MAIL_PASSWORD=rrnnucvnqlbsl MAIL_ENCRYPTION=tls MAIL_FROM_ADDRESS=mygoogle@gmail.com MAIL_FROM_NAME="${APP_NAME}"Step 2: Create Mail
In this step we will create mail class TestMail for email sending. Here we will write code for which view will call and object of user. So let's run bellow command.
php artisan make:mail TestMail
app/Mail/TestMail.php
<?php namespace App\Mail; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Mail\Mailable; use Illuminate\Queue\SerializesModels; class TestMail extends Mailable { use Queueable, SerializesModels; public $details; /** * Create a new message instance. * * @return void */ public function __construct($details) { $this->details = $details; } /** * Build the message. * * @return $this */ public function build() { return $this->subject('Mail from MyWebtuts.com') ->view('emails.TestMail',compact($details)); } } ?>Step 3: Create Blade View
In this step, we will create blade view file and write email that we want to send. now we just write some dummy text. create bellow files on "emails" folder.
resources/views/emails/TestMail.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel - How to send email?-MyWebtuts.com</title> </head> <body> <h1>{{ $details['title'] }}</h1> <p>{{ $details['body'] }}</p> <p>Thank you</p> </body> </html>Step 4: Add Route
Now at last we will create "TestMail" for sending our test email. so let's create bellow web route for testing send email.
routes/web.php
Route::get('send-mail', function () { $details = [ 'title' => 'Mail from MyWebtuts.com', 'body' => 'This is for testing email using smtp' ]; \Mail::to('your_receiver_email@gmail.com')->send(new \App\Mail\TestMail($details)); dd("Email is Sent."); });
Now you can run and check example, It will send you email, let' see.
php artisan serve
It will help you....