React Native Date Picker With Input Example
Oct 09, 2021 . Admin
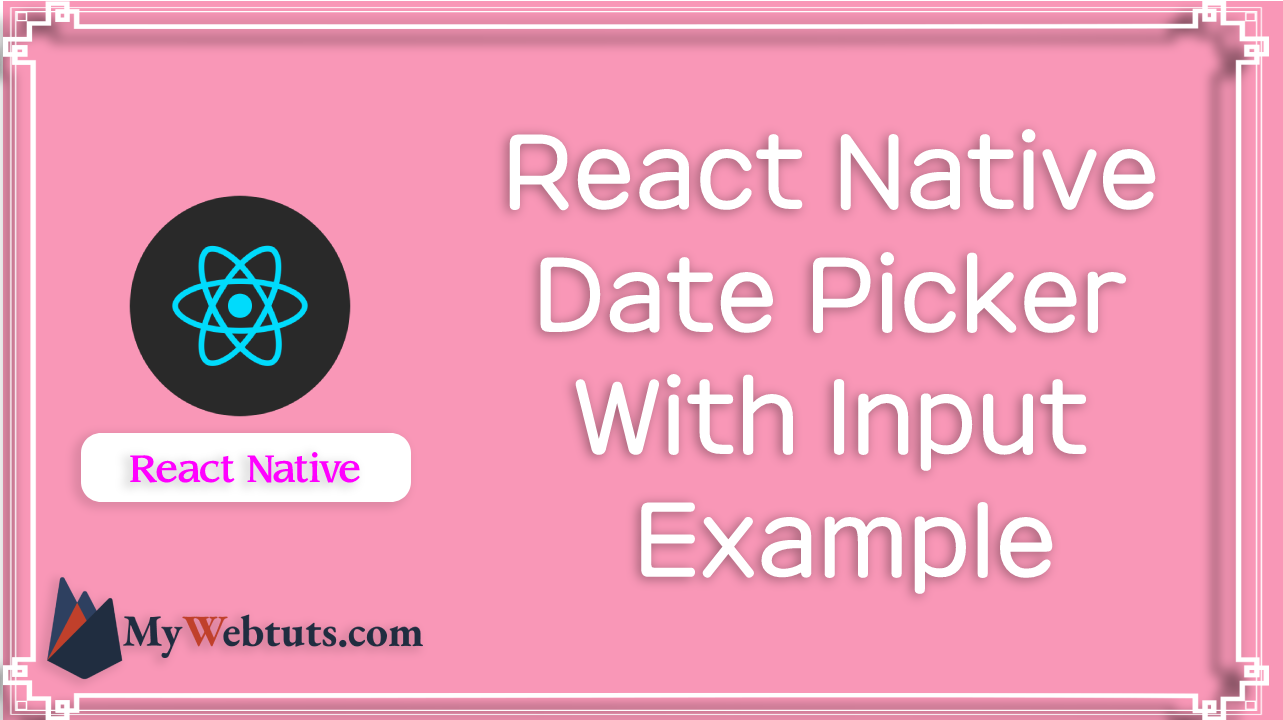
Hi Guys,
Today, I will learn you how to add date picker with input in react native. we will show example of react native date picker with input.You can easily implement date picker with input in react native. First i will import react-native-datepicker after I will implement date picker in react native.
Here, I will give you full example for date picker with input using react native as bellow.
Step 1 - Create projectIn the first step Run the following command for create project.
expo init MyWebtutsProjectStep 2 - Install Package
In the step,I will install react-native-datepicker package using yarn.
yarn add react-native-datepickerStep 3 - App.js
In this step, You will open App.js file and put the code.
import React, {useState} from 'react'; import { SafeAreaView, StyleSheet, Text, View } from 'react-native'; import DatePicker from 'react-native-datepicker'; const DatePickerApp = () => { const [date, setDate] = useState('09-10-2021'); return ( <SafeAreaView style={styles.container}> <View style={styles.container}> <Text style={styles.text}>Birth Date :</Text> <DatePicker style={styles.datePickerStyle} date={date} mode="date" placeholder="select date" format="DD/MM/YYYY" minDate="01-01-1900" maxDate="01-01-2000" confirmBtnText="Confirm" cancelBtnText="Cancel" customStyles={{ dateIcon: { position: 'absolute', right: -5, top: 4, marginLeft: 0, }, dateInput: { borderColor : "gray", alignItems: "flex-start", borderWidth: 0, borderBottomWidth: 1, }, placeholderText: { fontSize: 17, color: "gray" }, dateText: { fontSize: 17, } }} onDateChange={(date) => { setDate(date); }} /> </View> </SafeAreaView> ); }; export default DatePickerApp; const styles = StyleSheet.create({ container: { flex: 1, padding: 10, justifyContent: 'center', alignItems: 'center', backgroundColor : '#A8E9CA' }, title: { textAlign: 'left', fontSize: 20, fontWeight: 'bold', }, datePickerStyle: { width: 230, }, text: { textAlign: 'left', width: 230, fontSize: 16, color : "#000" } });Step 4 - Run project
In the last step run your project using bellow command.
expo startOutput
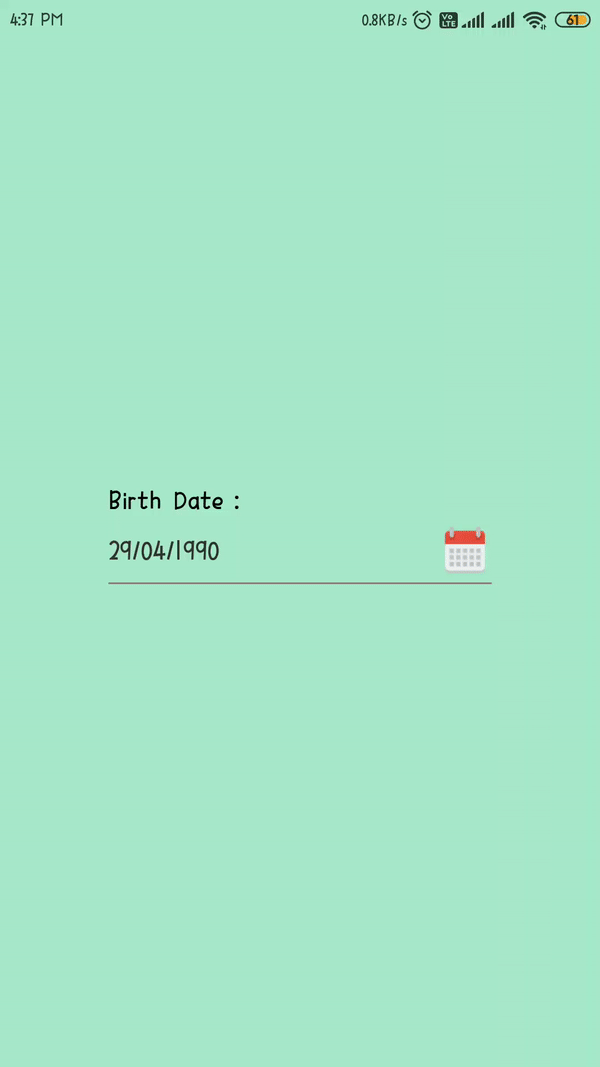
It will help you..