How To Use Flip Image with Animation Using React Native?
Nov 20, 2021 . Admin
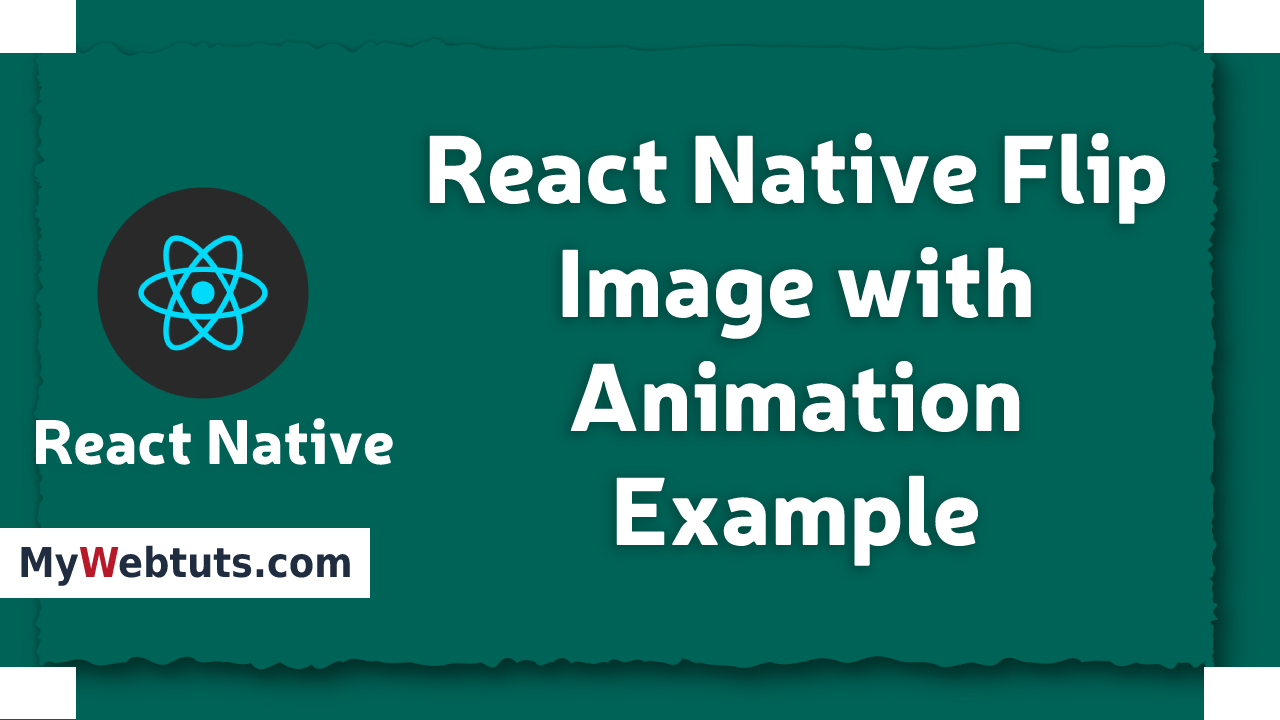
Hi Guys,
Today, I will learn you how to implement simple flip image with animation in react native. This is a short guide on use flip image with animation in react native. Let's get started with how to use flip image with animation in react native.
Here, I will give you full example for simply implement flip image using animation using react native as bellow.
Step 1 - Create projectIn the first step Run the following command for create project.
expo init MyWebtutsProjectStep 2 - App.js
In this step, You will open App.js file and put the code.
import React from 'react'; import { SafeAreaView, StyleSheet, View, Text, TouchableOpacity, Animated } from 'react-native'; const MyWebtutsProject = () => { let animatedValue = new Animated.Value(0); let currentValue = 0; animatedValue.addListener(({ value }) => { currentValue = value; }); const flipImageAnimation = () => { if (currentValue >= 90) { Animated.spring(animatedValue, { toValue: 0, tension: 10, friction: 8, useNativeDriver: false, }).start(); } else { Animated.spring(animatedValue, { toValue: 180, tension: 10, friction: 8, useNativeDriver: false, }).start(); } }; const setInterpolate = animatedValue.interpolate({ inputRange: [0, 180], outputRange: ['180deg', '360deg'], }); const rotateYAnimatedStyle = { transform: [{ rotateY: setInterpolate }], }; return ( <SafeAreaView style={{ flex: 1 }}> <View style={styles.container}> <Animated.Image source={{ uri: 'https://www.mywebtuts.com/image/imgpsh_fullsize.png', }} style={[rotateYAnimatedStyle, styles.imageStyle]} /> <TouchableOpacity style={styles.buttonStyle} onPress={flipImageAnimation}> <Text style={styles.buttonTextStyle}> Flip The Image </Text> </TouchableOpacity> </View> </SafeAreaView> ); }; const styles = StyleSheet.create({ container: { flex: 1, alignItems: 'center', justifyContent: 'center', }, buttonStyle: { backgroundColor: 'black', padding: 5, marginTop: 32, minWidth: 200, }, buttonTextStyle: { padding: 5, color: 'white', textAlign: 'center', }, imageStyle: { width: 150, height: 150, borderRadius: 6, }, }); export default MyWebtutsProject;Step 3 - Run project
In the last step run your project using bellow command.
expo startOutput
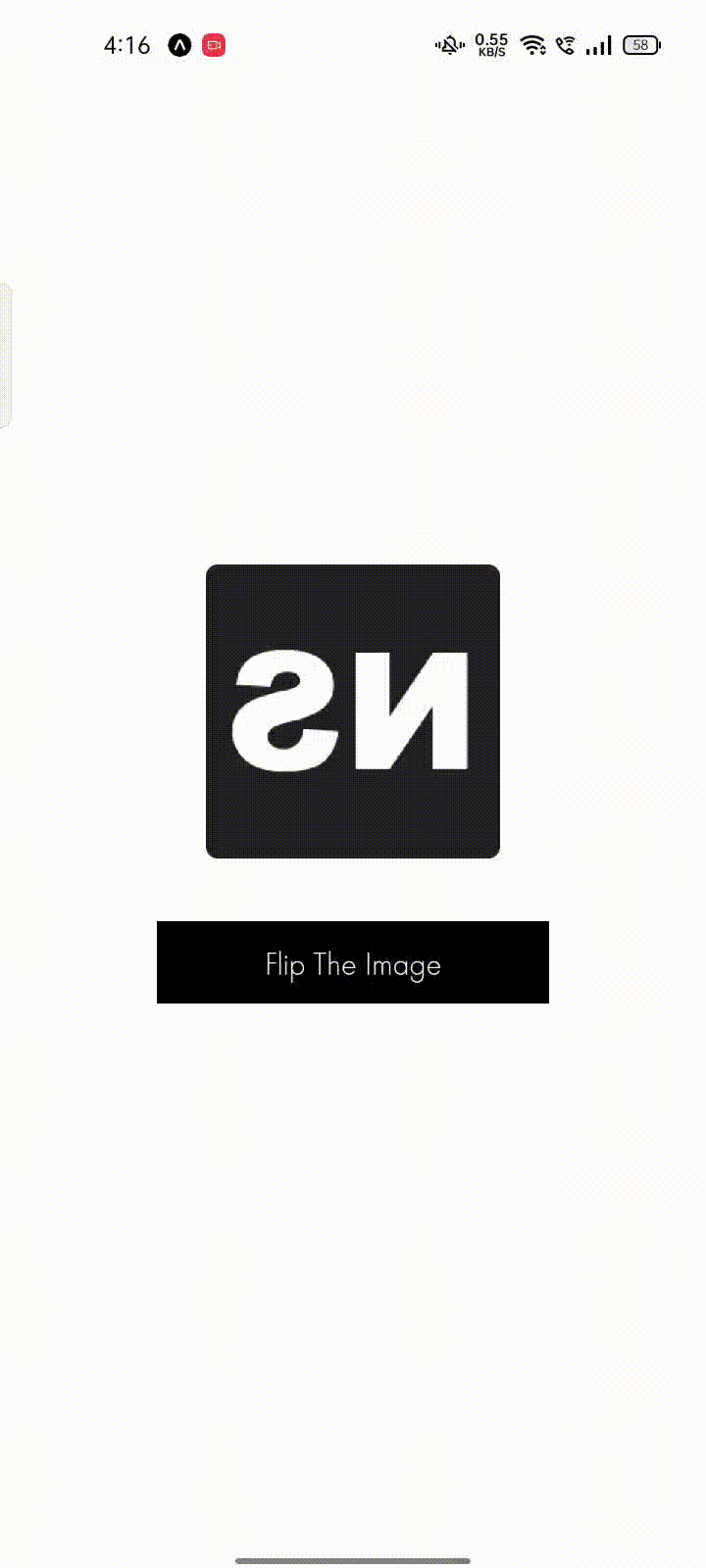
It will help you..